C++编写一个学生和教师输入和显示程序。 要求: (1)学生数据有编号、姓名、班号和成绩; (2)教师数据有编号、姓名、职称和部门; (3)根据共同数据特点,抽象一个person类,并作为学生和教师的基类。 (4)每个类均有构造函数和析构函数,实现学生和教师的数据输入,并显示结果。
时间: 2024-04-07 12:29:00 浏览: 43
好的,以下是C++代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Person {
public:
Person(int id, string name) : id(id), name(name) {}
virtual ~Person() {}
virtual void input() = 0;
virtual void display() = 0;
protected:
int id;
string name;
};
class Student : public Person {
public:
Student(int id, string name, int classNo, float score)
: Person(id, name), classNo(classNo), score(score) {}
void input() override {
cout << "请输入学生信息:" << endl;
cout << "学号:" << endl;
cin >> id;
cout << "姓名:" << endl;
cin >> name;
cout << "班级:" << endl;
cin >> classNo;
cout << "成绩:" << endl;
cin >> score;
}
void display() override {
cout << "学生信息:" << endl;
cout << "学号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "班级:" << classNo << endl;
cout << "成绩:" << score << endl;
}
private:
int classNo;
float score;
};
class Teacher : public Person {
public:
Teacher(int id, string name, string title, string department)
: Person(id, name), title(title), department(department) {}
void input() override {
cout << "请输入教师信息:" << endl;
cout << "工号:" << endl;
cin >> id;
cout << "姓名:" << endl;
cin >> name;
cout << "职称:" << endl;
cin >> title;
cout << "部门:" << endl;
cin >> department;
}
void display() override {
cout << "教师信息:" << endl;
cout << "工号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "职称:" << title << endl;
cout << "部门:" << department << endl;
}
private:
string title;
string department;
};
int main() {
Person* person[2];
person[0] = new Student(0, "", 0, 0);
person[1] = new Teacher(0, "", "", "");
for (int i = 0; i < 2; i++) {
person[i]->input();
person[i]->display();
}
delete person[0];
delete person[1];
return 0;
}
```
我们定义了一个Person类作为学生和教师的基类,其中包含了id和name两个属性,以及input和display两个虚函数。然后我们再分别定义了Student类和Teacher类,它们都继承自Person类,并实现了input和display函数。最后在main函数中创建了一个Person类型的数组,分别存放了一个Student对象和一个Teacher对象,然后循环调用它们的input和display函数,最后释放内存。
相关推荐
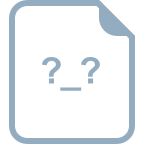
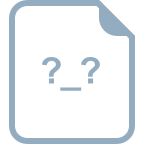













