用c#的系统的ziparchive类写一个解压带密码的zip压缩文件,带进度百分比
时间: 2024-09-18 11:07:46 浏览: 105
在C#中使用`System.IO.Compression.ZipFile`类可以方便地操作ZIP文件,包括解压带密码的压缩文件。这里有一个示例展示了如何解压带密码的ZIP文件并显示进度百分比:
```csharp
using System;
using System.IO;
using System.IO.Compression;
public void DecompressZipWithPassword(string sourcePath, string destinationPath, string password)
{
// 创建一个ZipArchive实例
using (var zipArchive = ZipFile.OpenRead(sourcePath))
{
// 检查是否存在密码保护
if (!zipArchive.IsEncrypted)
{
throw new Exception("The ZIP file is not encrypted.");
}
// 获取密码
var passwordBytes = Encoding.UTF8.GetBytes(password);
// 解压过程
foreach (var entry in zipArchive.Entries)
{
// 如果是文件而不是目录
if (entry.FullName.EndsWith("/"))
continue;
// 设置解压路径
var destinationSubfolder = Path.Combine(destinationPath, entry.Name);
// 创建解压路径(如果不存在)
Directory.CreateDirectory(destinationSubfolder);
// 使用密码解压
using (FileStream outputStream = File.Create(Path.Combine(destinationSubfolder, entry.FullName)))
{
using (var zipEntryStream = entry.Open())
{
var cryptoStream = new CryptoStream(zipEntryStream, new DESCryptoServiceProvider(), CryptoStreamMode.Read);
byte[] buffer = new byte[4096];
int bytesRead;
long totalBytesToRead = entry.Length;
while ((bytesRead = cryptoStream.Read(buffer, 0, buffer.Length)) > 0)
{
outputStream.Write(buffer, 0, bytesRead);
double progressPercentage = (double)outputStream.Position / totalBytesToRead * 100;
Console.WriteLine($"Decompressing: {entry.FullName} - Progress: {progressPercentage:F2}%");
}
}
}
}
}
}
// 调用方法时传入源文件路径、目标解压路径和密码
DecompressZipWithPassword(@"path\to\encrypted.zip", @"path\to\destination", "your-password");
```
阅读全文
相关推荐






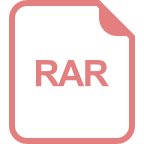





