用c#写一个创建zip压缩文件,带进度百分比
时间: 2024-09-23 08:09:53 浏览: 65
在C#中,你可以使用System.IO.Compression命名空间下的ZipArchive和ZipArchiveEntry类来创建ZIP压缩文件,并通过FileStream来跟踪进度百分比。下面是一个简单的示例:
```csharp
using System;
using System.IO;
using System.IO.Compression;
public class ZipProgressHandler : IAsyncResult {
private int completedPercentage;
public object AsyncState { get; set; }
public bool IsCompleted { get; set; }
public void UpdateProgress(int progress) {
Interlocked.Add(ref completedPercentage, progress);
// 更新UI或者触发事件来显示进度
OnProgressChanged(EventArgs.Empty);
}
public event EventHandler ProgressChanged;
protected virtual void OnProgressChanged(EventArgs e) {
if (ProgressChanged != null)
ProgressChanged(this, e);
}
}
public static void CreateZipFile(string sourceDirectory, string zipFileName, Action<ZipProgressHandler> onProgress = null) {
using var archive = new ZipArchive(zipFileName, ZipArchiveMode.Create, true); // true for overwrite existing files
// 获取源目录下所有文件和目录
var directoryInfo = new DirectoryInfo(sourceDirectory);
foreach (var fileInfo in directoryInfo.GetFiles()) {
AddToZip(archive, fileInfo.FullName, onProgress);
}
foreach (var dirInfo in directoryInfo.GetDirectories()) {
AddToZip(archive, dirInfo.FullName, onProgress);
}
// 添加完成回调处理进度
if (onProgress != null) {
var handler = new ZipProgressHandler();
handler.OnProgressChanged += onProgress(handler).UpdateProgress;
}
}
private static void AddToZip(ZipArchive archive, string filePath, Action<ZipProgressHandler> onProgress) {
ZipArchiveEntry entry;
try {
entry = archive.CreateEntry(filePath);
onProgress?.Invoke(handler => handler.UpdateProgress(50)); // 每添加一个文件更新进度为50%
File.Copy(filePath, entry.Open().FullName, true);
onProgress?.Invoke(handler => handler.UpdateProgress(100)); // 当文件复制完成后更新为100%
} catch (Exception ex) {
Console.WriteLine($"Error adding file to ZIP: {ex.Message}");
}
}
// 使用示例
CreateZipFile(@"C:\source\dir", @"C:\destination\output.zip");
```
在这个例子中,`CreateZipFile`函数接受源目录、输出ZIP文件名以及一个可选的进度处理委托。每添加一个文件到ZIP时,会调用传递的`onProgress`回调并更新进度。
阅读全文
相关推荐












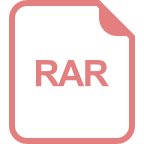





