用c#写一个压缩带密码的zip压缩文件,带进度百分比
时间: 2024-09-23 10:10:00 浏览: 52
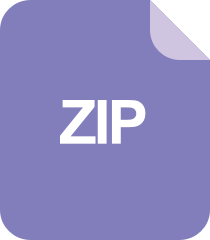
C#压缩/解压ZIP文件带密码功能

在 C# 中,你可以使用 `System.IO.Compression.ZipFile` 类以及一些额外的步骤来创建一个压缩文件,并添加密码保护。这里是一个简单的示例,展示如何完成这个任务:
```csharp
using System;
using System.IO;
using System.IO.Compression;
public class PasswordProtectedZip
{
private static void CompressWithPassword(string sourcePath, string destinationPath, string password)
{
using (FileStream sourceStream = new FileStream(sourcePath, FileMode.Open))
{
byte[] passwordBytes = Encoding.UTF8.GetBytes(password);
// 创建加密流
using (MemoryStream encryptedStream = new MemoryStream())
{
using (ICryptoTransform encryptor = ProtectedData.CreateEncryptor(new RijndaelManaged(), passwordBytes, DataProtectionScope.LocalMachine))
{
using (CryptoStream cs = new CryptoStream(encryptedStream, encryptor, CryptoStreamMode.Write))
{
ZipArchive archive = new ZipArchive(cs, ZipArchiveMode.Create, true);
ZipArchiveEntry entry = archive.CreateEntry(Path.GetFileName(sourcePath));
CopyStream(sourceStream, entry.Open());
}
}
// 将加密后的数据写入到新的压缩文件中
File.WriteAllBytes(destinationPath, encryptedStream.ToArray());
}
}
}
public static void CopyStream(Stream source, Stream destination)
{
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = source.Read(buffer, 0, buffer.Length)) > 0)
{
destination.Write(buffer, 0, bytesRead);
}
}
public static void Main(string[] args)
{
string sourceFilePath = "source_file.txt";
string zipFilePath = "password_protected.zip";
string password = "example_password";
try
{
Console.WriteLine("Compressing file...");
CompressWithPassword(sourceFilePath, zipFilePath, password);
Console.WriteLine($"Compression complete! {zipFilePath} is now encrypted with a password.");
}
catch (Exception ex)
{
Console.WriteLine($"Error occurred: {ex.Message}");
}
阅读全文
相关推荐
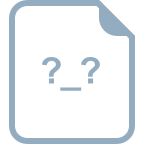
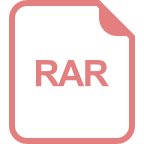









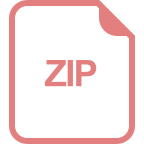
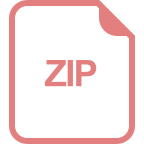
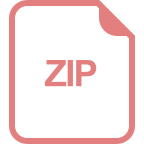
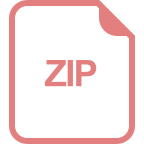
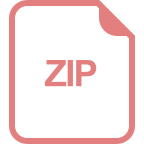