项目运行发生如文件中的报错,应该如何解决
时间: 2024-12-24 15:41:14 浏览: 5
从日志中可以看出,项目启动失败的原因是 `User` 实体类未被管理(`Not a managed type: class com.example.wiki.entity.User`)。这通常是因为以下几个原因之一:
1. **缺少 `@Entity` 注解**:确保 `User` 类上有 `@Entity` 注解,这样 JPA 才能识别它为一个实体类。
2. **包扫描配置错误**:确保 `@SpringBootApplication` 或 `@EntityScan` 注解正确地指定了包含 `User` 实体类的包路径。
3. **依赖缺失或版本不匹配**:确保所有必要的 JPA 和 Spring Data JPA 依赖都已正确添加,并且版本兼容。
### 解决步骤
#### 1. 检查 `User` 类
确保 `User` 类上有 `@Entity` 注解:
```java
package com.example.wiki.entity;
import jakarta.persistence.Entity;
import jakarta.persistence.GeneratedValue;
import jakarta.persistence.GenerationType;
import jakarta.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
// 其他字段和方法
}
```
#### 2. 检查包扫描配置
确保 `@SpringBootApplication` 注解所在的主类位于正确的包路径下,或者显式指定 `@EntityScan` 注解:
```java
package com.example.wiki;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.data.jpa.repository.config.EnableJpaRepositories;
@SpringBootApplication
@EnableJpaRepositories("com.example.wiki.repository")
@EntityScan("com.example.wiki.entity")
public class WikiApplication {
public static void main(String[] args) {
SpringApplication.run(WikiApplication.class, args);
}
}
```
#### 3. 检查依赖
确保 `pom.xml` 文件中有以下依赖:
```xml
<dependencies>
<!-- Spring Boot Starter Data JPA -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- MySQL Connector -->
<dependency>
<groupId>com.mysql</groupId>
<artifactId>mysql-connector-j</artifactId>
</dependency>
<!-- 其他必要依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<scope>provided</scope>
</dependency>
</dependencies>
```
#### 4. 检查 `UserRepository` 接口
确保 `UserRepository` 接口继承了 `JpaRepository` 并且包路径正确:
```java
package com.example.wiki.repository;
import com.example.wiki.entity.User;
import org.springframework.data.jpa.repository.JpaRepository;
public interface UserRepository extends JpaRepository<User, Long> {
}
```
#### 5. 检查 `UserService` 类
确保 `UserService` 类正确注入了 `UserRepository`:
```java
package com.example.wiki.service;
import com.example.wiki.entity.User;
import com.example.wiki.repository.UserRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
// 业务逻辑方法
}
```
#### 6. 检查 `UserController` 类
确保 `UserController` 类正确注入了 `UserService`:
```java
package com.example.wiki.controller;
import com.example.wiki.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/users")
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
// 控制器方法
}
```
### 总结
1. 确保 `User` 类上有 `@Entity` 注解。
2. 确保 `@SpringBootApplication` 或 `@EntityScan` 注解正确指定了包含 `User` 实体类的包路径。
3. 确保 `pom.xml` 中有必要的 JPA 和数据库连接依赖。
4. 确保 `UserRepository` 接口继承了 `JpaRepository`。
5. 确保 `UserService` 类正确注入了 `UserRepository`。
6. 确保 `UserController` 类正确注入了 `UserService`。
通过以上步骤,应该可以解决 `Not a managed type` 的问题。如果问题仍然存在,请检查是否有其他配置或代码问题。
阅读全文
相关推荐
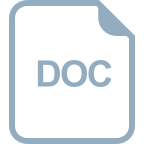
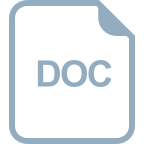
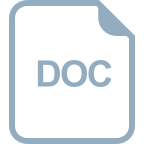
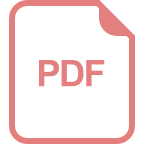
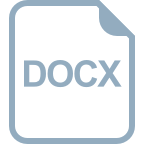
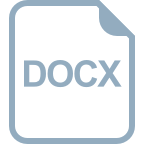
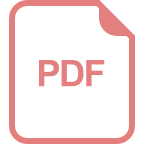
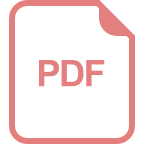
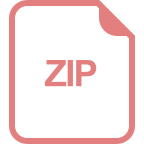
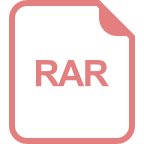
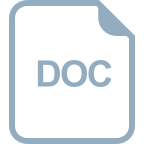
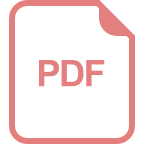
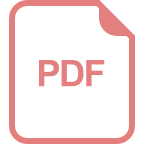
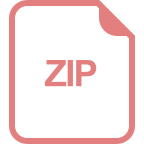




