RSA密码算法Python实现
时间: 2024-10-23 09:11:14 浏览: 29
RSA(Rivest-Shamir-Adleman)是一种非对称加密算法,常用于数据安全传输和数字签名。在Python中,你可以使用内置的`cryptography`库来实现RSA算法。以下是基本步骤:
首先,你需要安装`cryptography`库,可以使用pip安装:
```bash
pip install cryptography
```
然后,这里是简单的RSA算法实现:
```python
from cryptography.hazmat.primitives import hashes
from cryptography.hazmat.primitives.asymmetric import rsa
from cryptography.hazmat.backends import default_backend
from cryptography.hazmat.primitives.asymmetric import padding
# 生成公钥和私钥
def generate_keys():
private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048,
backend=default_backend()
)
public_key = private_key.public_key()
return private_key, public_key
# 加密消息
def encrypt(message, public_key):
ciphertext = public_key.encrypt(
message.encode(),
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
return ciphertext
# 解密消息
def decrypt(ciphertext, private_key):
plaintext = private_key.decrypt(
ciphertext,
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
return plaintext.decode()
# 示例
private_key, public_key = generate_keys()
message = "Hello, RSA!"
encrypted_message = encrypt(message, public_key)
decrypted_message = decrypt(encrypted_message, private_key)
print(f"Original message: {message}")
print(f"Encrypted message: {encrypted_message}")
print(f"Decrypted message: {decrypted_message}")
```
注意,实际应用中需要妥善保管私钥,因为它是解密的关键。
阅读全文
相关推荐
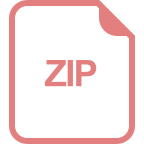










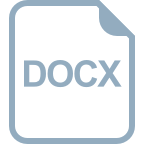
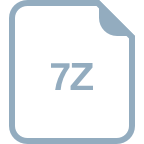