c语言使用堆栈方法输出从源点到每个终点的最短路径及最短路径长度
时间: 2024-10-29 17:11:38 浏览: 5
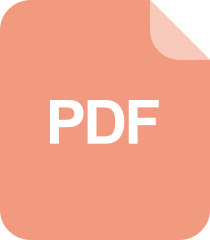
python实现最短路径的实例方法
在C语言中,利用堆栈数据结构寻找从源点到所有终点的最短路径通常涉及到Dijkstra算法或Floyd-Warshall算法,特别是广度优先搜索(BFS)在有向无环图(DAG)或无向图中非常适合。这里以BFS为例,因为它可以找到单源最短路径:
首先,你需要一个邻接矩阵或邻接表来存储图的结构,源点通常是0或某个特定值。
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTICES 100 // 假设最多100个顶点
// 堆栈操作函数
void push(int data[], int top) {
data[top] = data[current];
current++;
}
int pop() {
return data[--current];
}
// 带权重的邻接列表表示法(假设weight数组存储边的权重)
int* graph[MAX_VERTICES], weight[MAX_VERTICES][MAX_VERTICES];
void bfs(int src) {
int distance[MAX_VERTICES], visited[MAX_VERTICES] = {0};
int top = -1;
distance[src] = 0;
push(distance, top++);
while (top != -1) {
int u = pop();
printf("Current vertex %d with shortest path length: %d\n", u, distance[u]);
// 遍历u的所有邻居
for (int v = 0; v < MAX_VERTICES; ++v) {
if (!visited[v] && distance[u] + weight[u][v] < distance[v]) {
distance[v] = distance[u] + weight[u][v];
push(distance, top);
visited[v] = 1;
}
}
}
}
int main() {
int vertices, start_vertex;
// 初始化并输入图的数据和起点
scanf("%d %d", &vertices, &start_vertex);
// ... 具体填充图的结构 ...
bfs(start_vertex);
return 0;
}
```
在这个例子中,`bfs`函数会按照源点开始,遍历每个节点,更新其到达源点的最短路径长度,并记录路径。当你运行程序时,它将打印出从给定的源点到各个终点的最短路径及其长度。
阅读全文
相关推荐
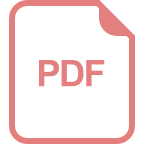
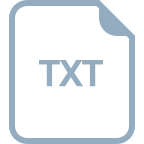

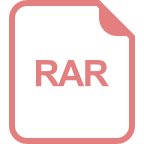
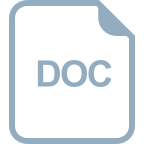








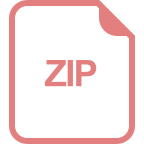
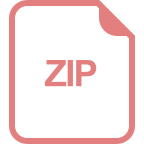