1.输入有向图,并存储。 2.实现最短路径算法,最短路径算法的实现,编写算法对带权有向图中从源点到其他各点的最短路径。 用c语言完成以上实验要求,并给出代码
时间: 2024-12-02 09:22:05 浏览: 25
在C语言中,我们可以使用迪杰斯特拉(Dijkstra's)算法或者Floyd-Warshall算法来求解有向图的最短路径。这里以Dijkstra算法为例,因为它通常用于单源最短路径的问题。
首先,我们需要定义结构体来表示图的节点和边:
```c
#include <stdio.h>
#include <limits.h>
typedef struct Node {
int id;
int distance; // 到源点的距离
int previous; // 指向前一个最近节点的索引
} Node;
typedef struct Edge {
int source;
int destination;
int weight;
} Edge;
```
然后实现函数来读取图的信息、初始化距离数组以及进行Dijkstra算法:
```c
void read_graph(Edge edges[], int num_edges, Node nodes[], int num_nodes);
void dijkstra(Node graph[], int start_node, int num_nodes);
```
`read_graph`函数负责读取输入的有向图的边,`dijkstra`函数则是Dijkstra算法的核心部分,包括优先队列的操作、更新节点距离等步骤。
Dijkstra算法伪代码:
```c
void dijkstra(Node graph[], int start_node, int num_nodes) {
for (int i = 0; i < num_nodes; i++) {
graph[i].distance = INT_MAX;
graph[i].previous = -1;
}
graph[start_node].distance = 0;
// 创建优先队列并插入起始节点
priority_queue<Node*, vector<Node*>, greater<Node*>> pq;
pq.push(&graph[start_node]);
while (!pq.empty()) {
Node* current = pq.top();
pq.pop();
if (current->distance != INT_MAX) {
for (Edge edge : edges) {
if (edge.source == current->id && edge.weight + current->distance < graph[edge.destination].distance) {
graph[edge.destination].distance = edge.weight + current->distance;
graph[edge.destination].previous = current->id;
pq.push(&graph[edge.destination]);
}
}
}
}
}
```
最后,你可以通过调用这个函数并传入起点和节点数来计算最短路径:
```c
int main() {
// 初始化图信息
Edge edges[] = {/* 图的具体边 */};
int num_edges = sizeof(edges) / sizeof(Edge);
Node nodes[num_nodes];
read_graph(edges, num_edges, nodes, num_nodes);
int source_node = /* 输入的源点 */;
dijkstra(nodes, source_node, num_nodes);
printf("源点 %d 到其他点的最短路径:\n", source_node);
for (int i = 0; i < num_nodes; i++) {
if (nodes[i].distance != INT_MAX) {
printf("到节点 %d 的最短路径长度为: %d\n", i, nodes[i].distance);
}
}
return 0;
}
```
阅读全文
相关推荐
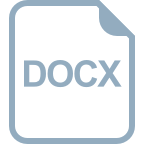
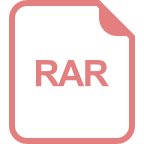
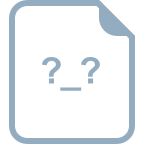




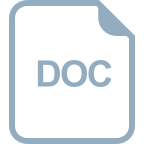
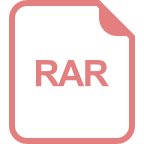
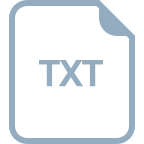
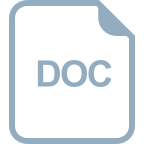
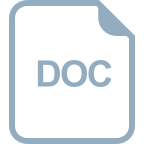
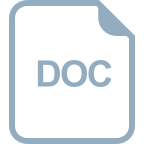





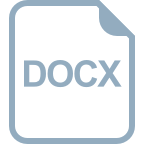