Python发文件给企业微信
时间: 2024-09-04 16:00:21 浏览: 70
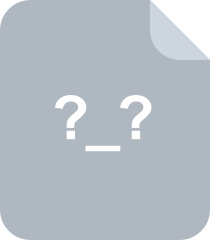
python+排班+企业微信定时发送通知
在Python中,你可以使用第三方库如`flask-wechaty`来发送文件到企业微信。首先,你需要安装所需库:
```bash
pip install flask wechaty flasgger requests
```
然后,你可以编写一个简单的脚本来实现这个功能,这里是一个基本的例子:
```python
from flask import Flask, request, jsonify
import wechaty
app = Flask(__name__)
# 初始化企业微信机器人
def init_wechaty():
bot = wechaty.WebSocketBot(
'your-bot-token', # 请替换为你自己的bot token
'your-bot-secret', # 请替换为你自己的bot secret
host='your-bot-host' # 请替换为企业微信API的host地址,默认是wss://qyapi.weixin.qq.com/webhook
)
return bot
bot = init_wechaty()
@app.route('/upload', methods=['POST'])
def upload_file():
file = request.files['file']
if not file:
return jsonify({"error": "No file uploaded."}), 400
# 使用企业微信机器人的message事件处理器发送文件
async def handle_message(msg):
await msg.say(f"收到了您的文件:{file.filename}")
await msg.send(file.stream.read(), file_type=file.content_type)
bot.on('message', handle_message)
try:
with open(file.filename, 'rb') as f:
await bot.privateRoom('room-id').send(file.stream.read(), file_type=file.content_type) # 请替换为实际的企业微信群ID
return jsonify({"success": True, "filename": file.filename})
except Exception as e:
print(f"Error occurred: {e}")
return jsonify({"error": str(e)}), 500
if __name__ == '__main__':
app.run(debug=True)
```
在这个例子中,你需要将`your-bot-token`、`your-bot-secret`以及`room-id`替换为你的实际值。当用户通过HTTP POST请求提交文件时,文件会被处理并发送到指定的企业微信群。
阅读全文
相关推荐
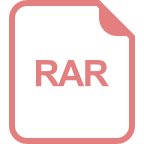
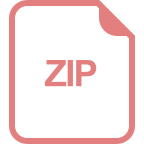
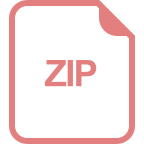
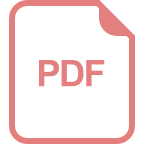













