C语言用栈进制转换(完整代码)
时间: 2024-10-12 22:01:47 浏览: 61
在C语言中,将十进制数转换成栈上表示的另一种进制(如二进制、八进制或十六进制),可以借助循环和位操作来实现。下面是一个简单的示例,展示如何将十进制整数转换为二进制并存储到栈上:
```c
#include <stdio.h>
#include <stdlib.h>
// Stack structure and functions
typedef struct {
int data[10]; // Assuming a stack of size 10 for simplicity
int top;
} Stack;
Stack* createStack() {
Stack *stack = (Stack*)malloc(sizeof(Stack));
stack->top = -1;
return stack;
}
void push(Stack *stack, int value) {
if (stack->top >= 9) {
printf("Stack overflow!\n");
return;
}
stack->data[++stack->top] = value;
}
int pop(Stack *stack) {
if (stack->top == -1) {
printf("Stack underflow!\n");
return -1; // Return an error code or some sentinel value
}
return stack->data[stack->top--];
}
// Function to convert decimal to binary
void decimalToBinary(int num, Stack *stack) {
while (num > 0) {
push(stack, num % 2); // Push the remainder onto the stack
num /= 2;
}
}
// Print the contents of the stack as binary
void printStackAsBinary(Stack *stack) {
int i;
for (i = stack->top; i >= 0; i--) {
printf("%d", stack->data[i]);
}
printf("\n");
}
int main() {
Stack *binaryStack = createStack();
int decimalNum = 42;
decimalToBinary(decimalNum, binaryStack);
printStackAsBinary(binaryStack);
free(binaryStack);
return 0;
}
```
在这个例子中,`decimalToBinary`函数会把给定的十进制数`num`转换为它的二进制形式,并通过不断地除以2和取余的方式,将结果逐个压入栈中。最后,`printStackAsBinary`函数用于显示栈中储存的二进制数字。
阅读全文
相关推荐
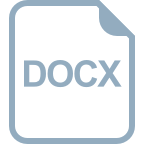
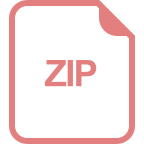
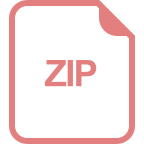




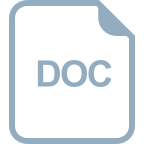










