通过c语言数据栈实现十进制转换为二进制
时间: 2023-11-21 17:04:22 浏览: 71
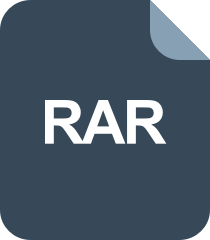
用栈实现十进制转换成二进制(c语言)

以下是使用c语言数据栈实现十进制转换为二进制的代码:
```c
#include <stdio.h>
#define MAX_STACK_SIZE 100
int stack[MAX_STACK_SIZE];
int top = -1;
void push(int data) {
if (top >= MAX_STACK_SIZE - 1) {
printf("Stack Overflow!\n");
return;
}
stack[++top] = data;
}
int pop() {
if (top == -1) {
printf("Stack Underflow!\n");
return -1;
}
return stack[top--];
}
int is_empty() {
return top == -1;
}
void decimal_to_binary(int decimal) {
if (decimal == 0) {
push(0);
}
while (decimal > 0) {
int remainder = decimal % 2;
push(remainder);
decimal /= 2;
}
printf("Binary representation: ");
while (!is_empty()) {
printf("%d", pop());
}
printf("\n");
}
int main() {
int decimal;
printf("Enter a decimal number: ");
scanf("%d", &decimal);
decimal_to_binary(decimal);
return 0;
}
```
首先,定义了一个数据栈 `stack` 和栈顶指针 `top`,同时定义了 `MAX_STACK_SIZE` 作为栈的最大容量。接着,实现了 `push`、`pop` 和 `is_empty` 函数,分别用于将数据压入栈中、从栈中弹出数据和判断栈是否为空。
在 `decimal_to_binary` 函数中,首先判断输入的十进制数是否为 0,如果是,就将 0 压入栈中。然后,对于非 0 的十进制数,依次将其除以 2,取余数,并将余数压入栈中。最后,输出栈中元素,即为二进制表示形式。注意,栈是先进后出的数据结构,因此输出时需要倒序输出。
在 `main` 函数中,首先提示用户输入十进制数,然后调用 `decimal_to_binary` 函数进行转换。
阅读全文
相关推荐
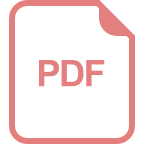
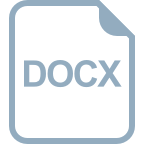
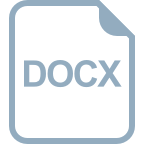



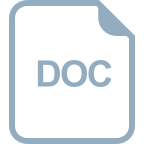







