Turns off the ESP32-CAM white on-board LED (flash) connected to GPIO 4
时间: 2024-10-27 13:11:45 浏览: 17
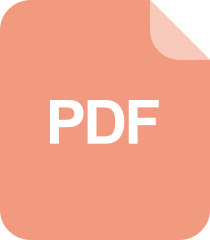
藏经阁-SS7-Attacker-Heaven-Turns-Into-Riot-How-To-Make-Nation-State
ESP32-CAM是一款基于ESP32芯片并集成了摄像头功能的开发板,它通常有一个白色的LED指示灯连接到GPIO 4作为电源状态指示。如果你想关闭这个LED,你需要通过编程操作来控制对应的GPIO管脚。在ESP32的框架中,如Espressif IoT SDK,你可以使用`digitalWrite()`函数来控制GPIO的输出状态。
首先,确保你已经初始化了GPIO 4,并将其设置为输入或输出模式。然后,可以编写类似下面的代码:
```cpp
#include <WiFi.h>
#include <ESP32CAM.h>
void setup() {
// 初始化摄像头模块
Camera.begin();
// 如果GPIO 4默认是输出模式,设置为低电平(关闭LED)
pinMode(GPIO4, OUTPUT); // 假设GPIO4的宏定义是4
digitalWrite(GPIO4, LOW);
}
void loop() {
// 主程序循环
}
```
在这个例子中,`digitalWrite(GPIO4, LOW)`会将LED设置为关闭状态,即LED处于低电平,不会发光。记得替换`GPIO4`为实际的GPIO编号。
阅读全文
相关推荐
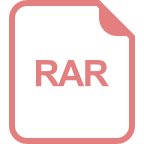
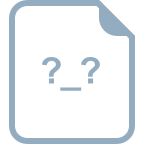
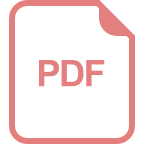
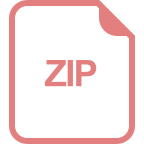
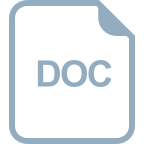
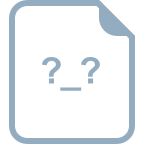
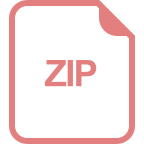









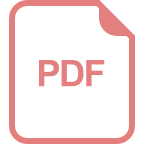
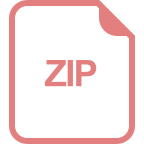