顺序表的编程实现与测试 基本要求:成功编写顺序表类的类体和类成员函数;编写main()函数对class SeqList进行测试,要求使用菜单选择各项功能。实现线性表的遍历、按位查找、按值查找、插入、删除等操作
时间: 2024-10-06 16:03:29 浏览: 34
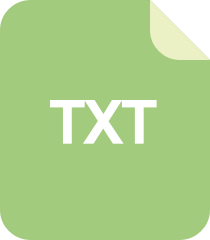
顺序表的实现
顺序表(如数组)是一种基础的数据结构,其主要思想是将元素存储在连续的内存空间中。下面是一个简单的顺序表类的实现,包括了基本的操作如遍历、查找和修改:
```cpp
#include <iostream>
// 定义顺序列表节点
template <typename T>
struct ListNode {
T value;
ListNode* next;
};
// 顺序列表类
template <typename T>
class SeqList {
public:
// 构造函数,初始化为空列表
SeqList() : head(nullptr), size(0) {}
// 长度获取
int size() const { return size; }
// 插入元素到列表末尾
void insert(T val) {
if (size == capacity) expandCapacity();
ListNode<T>* newNode = new ListNode<T>{val, nullptr};
newNode->next = head;
head = newNode;
size++;
}
// 删除指定位置的元素
void remove(int index) {
if (index >= size || index < 0) return;
ListNode<T>* current = head;
for (int i = 0; i < index && current; ++i)
current = current->next;
if (current) {
ListNode<T>* temp = current->next;
delete current;
head = temp;
size--;
}
}
// 查找元素
bool find(T val) const {
ListNode<T>* node = head;
while (node) {
if (node->value == val) return true;
node = node->next;
}
return false;
}
private:
// 遍历列表
void traverse() const {
ListNode<T>* node = head;
std::cout << "Elements: ";
while (node) {
std::cout << node->value << " ";
node = node->next;
}
std::cout << std::endl;
}
// 扩展容量
void expandCapacity() {
capacity *= 2;
ListNode<T>** oldList = &head;
ListNode<T>** newList = new ListNode<T>*[capacity];
for (int i = 0; i < size; ++i) {
newList[i] = oldList[i];
}
delete[] oldList;
head = newList;
capacity--;
}
ListNode<T>* head;
int size;
int capacity; // 默认初始容量
};
// 主函数测试
int main() {
SeqList<int> list;
int choice;
do {
std::cout << "\nChoose an operation:\n"
<< "1. Insert\n"
<< "2. Remove\n"
<< "3. Find\n"
<< "4. Traverse\n"
<< "5. Exit\n";
std::cin >> choice;
switch (choice) {
case 1:
int value;
std::cout << "Enter a value to insert: ";
std::cin >> value;
list.insert(value);
break;
case 2:
int index;
std::cout << "Enter index to remove: ";
std::cin >> index;
list.remove(index);
break;
case 3:
int searchValue;
std::cout << "Search for a value: ";
std::cin >> searchValue;
if (list.find(searchValue)) {
std::cout << "Found!\n";
} else {
std::cout << "Not found.\n";
}
break;
case 4:
list.traverse();
break;
case 5:
break;
default:
std::cout << "Invalid choice.\n";
}
} while (choice != 5);
return 0;
}
```
在这个例子中,我们创建了一个顺序列表模板类SeqList,实现了基本的插入、删除、查找和遍历功能。主函数通过菜单形式交互进行操作。
阅读全文
相关推荐
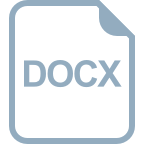
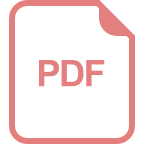















