用C语言编写:创建一个有向图G,以十字链表形式存储在内存中,试写一个判断该有向图中是否有环路(回路)的算法。
时间: 2024-04-16 20:26:33 浏览: 125
好的,我可以帮你解决这个问题。首先,我们需要定义有向图的数据结构。我们可以使用十字链表来表示有向图。下面是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义有向图的顶点结构
typedef struct Vertex {
int data; // 顶点数据
struct ArcNode* first_in; // 指向该顶点的入边表的第一个结点
struct ArcNode* first_out; // 指向该顶点的出边表的第一个结点
} Vertex;
// 定义有向图的边结点结构
typedef struct ArcNode {
struct Vertex* tail_vertex; // 指向弧尾顶点
struct Vertex* head_vertex; // 指向弧头顶点
struct ArcNode* next_in; // 指向下一条入边
struct ArcNode* next_out; // 指向下一条出边
} ArcNode;
// 创建有向图
void createGraph(Vertex** graph, int num_vertices) {
*graph = (Vertex*)malloc(num_vertices * sizeof(Vertex));
for (int i = 0; i < num_vertices; i++) {
(*graph)[i].data = i;
(*graph)[i].first_in = NULL;
(*graph)[i].first_out = NULL;
}
}
// 添加有向边
void addArc(Vertex* graph, int tail, int head) {
ArcNode* arc = (ArcNode*)malloc(sizeof(ArcNode));
arc->tail_vertex = &graph[tail];
arc->head_vertex = &graph[head];
arc->next_in = graph[head].first_in;
graph[head].first_in = arc;
arc->next_out = graph[tail].first_out;
graph[tail].first_out = arc;
}
// 判断有向图中是否存在环路
int hasCycle(Vertex* graph, int num_vertices) {
int* visited = (int*)malloc(num_vertices * sizeof(int));
for (int i = 0; i < num_vertices; i++) {
visited[i] = 0;
}
for (int i = 0; i < num_vertices; i++) {
if (!visited[i]) {
if (hasCycleUtil(graph, visited, i)) {
free(visited);
return 1;
}
}
}
free(visited);
return 0;
}
int hasCycleUtil(Vertex* graph, int* visited, int vertex) {
visited[vertex] = 1;
ArcNode* arc = graph[vertex].first_out;
while (arc != NULL) {
int next_vertex = arc->head_vertex->data;
if (!visited[next_vertex] && hasCycleUtil(graph, visited, next_vertex)) {
return 1;
} else if (visited[next_vertex]) {
return 1;
}
arc = arc->next_out;
}
visited[vertex] = 0;
return 0;
}
int main() {
int num_vertices = 4;
Vertex* graph;
createGraph(&graph, num_vertices);
addArc(graph, 0, 1);
addArc(graph, 1, 2);
addArc(graph, 2, 3);
addArc(graph, 3, 1); // 添加一个回路
int has_cycle = hasCycle(graph, num_vertices);
if (has_cycle) {
printf("The graph has a cycle.\n");
} else {
printf("The graph does not have a cycle.\n");
}
return 0;
}
```
这段代码创建了一个有向图,并检查图中是否存在环路。我们可以在 `main` 函数中修改 `num_vertices` 和 `addArc` 函数调用来适应不同的图。如果有环路存在,则输出 "The graph has a cycle.",否则输出 "The graph does not have a cycle."。
希望这能帮到你!如果你还有其他问题,请随时提问。
阅读全文
相关推荐


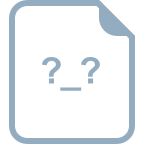
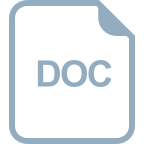
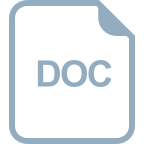
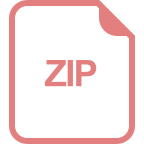
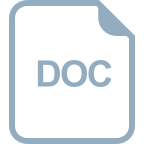
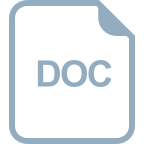

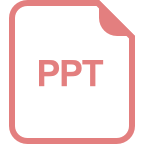
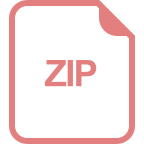
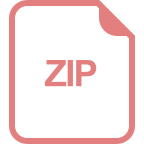
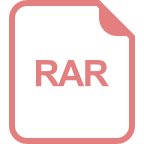
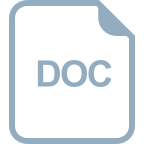
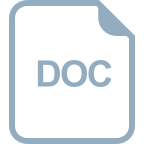
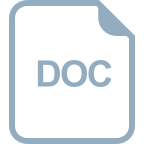