C语言数据结构以邻接表的形式创建一个无向图
时间: 2023-11-24 11:06:56 浏览: 122
下面是使用邻接表创建无向图的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义邻接表中的边结构体
typedef struct edge {
int dest; // 目标顶点
struct edge* next; // 下一条边
} Edge;
// 定义邻接表中的顶点结构体
typedef struct vertex {
Edge* edges; // 与该顶点相连的边
} Vertex;
// 创建无向图的邻接表
Vertex* createGraph(int numVertices, int numEdges, int* edges) {
Vertex* graph = (Vertex*)malloc(numVertices * sizeof(Vertex)); // 创建顶点数组
// 初始化每个顶点的边链表为空
for (int i = 0; i < numVertices; i++) {
graph[i].edges = NULL;
}
// 添加每条边到对应的顶点的边链表中
for (int i = 0; i < numEdges; i++) {
int src = edges[2*i];
int dest = edges[2*i+1];
// 添加 src -> dest 的边
Edge* edge1 = (Edge*)malloc(sizeof(Edge));
edge1->dest = dest;
edge1->next = graph[src].edges;
graph[src].edges = edge1;
// 添加 dest -> src 的边
Edge* edge2 = (Edge*)malloc(sizeof(Edge));
edge2->dest = src;
edge2->next = graph[dest].edges;
graph[dest].edges = edge2;
}
return graph;
}
// 打印邻接表表示的无向图
void printGraph(Vertex* graph, int numVertices) {
for (int i = 0; i < numVertices; i++) {
printf("%d: ", i);
Edge* edge = graph[i].edges;
while (edge != NULL) {
printf("%d ", edge->dest);
edge = edge->next;
}
printf("\n");
}
}
int main() {
int numVertices = 5;
int numEdges = 6;
int edges[] = {0, 1, 0, 2, 1, 2, 2, 3, 3, 4, 4, 0};
Vertex* graph = createGraph(numVertices, numEdges, edges);
printGraph(graph, numVertices);
return 0;
}
```
在上述代码中,我们先定义了邻接表中的边和顶点结构体,然后编写了`createGraph`函数来创建无向图的邻接表。在`createGraph`函数中,我们首先创建了一个大小为`numVertices`的顶点数组,然后初始化每个顶点的边链表为空。接着,我们遍历每条边,并将其添加到对应的顶点的边链表中。由于这是无向图,所以我们需要同时添加两条边:`src -> dest`和`dest -> src`。
最后,我们编写了`printGraph`函数来打印邻接表表示的无向图。在该函数中,我们遍历每个顶点,并打印它的编号以及与之相连的所有顶点的编号。
阅读全文
相关推荐





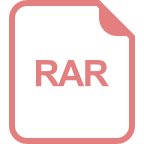












