用C语言建立一个以邻接表形式存储的图
时间: 2024-02-13 07:59:38 浏览: 65
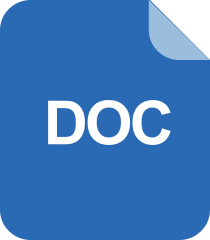
以邻接表的形式建立和存储图

好的,以下是用C语言建立一个以邻接表形式存储的图的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 边节点结构体
typedef struct EdgeNode {
int adjvex; // 邻接点在顶点数组中的下标
int weight; // 边的权值
struct EdgeNode *next; // 指向下一个边节点的指针
} EdgeNode;
// 顶点节点结构体
typedef struct VertexNode {
int data; // 顶点的数据
EdgeNode *first_edge; // 指向第一条依附于该顶点的边的指针
} VertexNode;
// 图结构体
typedef struct Graph {
VertexNode *vex; // 存储顶点的数组
int vex_num; // 顶点数
int edge_num; // 边数
} Graph;
// 初始化图
void init_graph(Graph *graph, int vex_num, int edge_num) {
graph->vex_num = vex_num;
graph->edge_num = edge_num;
graph->vex = (VertexNode *)malloc(sizeof(VertexNode) * vex_num);
for (int i = 0; i < vex_num; i++) {
graph->vex[i].data = i; // 顶点的数据为下标
graph->vex[i].first_edge = NULL; // 指向第一条边的指针初始化为 NULL
}
}
// 添加边
void add_edge(Graph *graph, int start, int end, int weight) {
EdgeNode *edge = (EdgeNode *)malloc(sizeof(EdgeNode));
edge->adjvex = end;
edge->weight = weight;
edge->next = graph->vex[start].first_edge;
graph->vex[start].first_edge = edge;
}
// 打印邻接表
void print_graph(Graph *graph) {
for (int i = 0; i < graph->vex_num; i++) {
printf("%d -> ", i);
EdgeNode *edge = graph->vex[i].first_edge;
while (edge != NULL) {
printf("%d(%d) ", edge->adjvex, edge->weight);
edge = edge->next;
}
printf("\n");
}
}
// 测试
int main() {
Graph graph;
int vex_num = 5, edge_num = 7;
init_graph(&graph, vex_num, edge_num);
add_edge(&graph, 0, 1, 1);
add_edge(&graph, 0, 2, 2);
add_edge(&graph, 1, 2, 3);
add_edge(&graph, 1, 3, 4);
add_edge(&graph, 2, 3, 5);
add_edge(&graph, 2, 4, 6);
add_edge(&graph, 3, 4, 7);
print_graph(&graph);
return 0;
}
```
这段代码实现了一个无向图,可以通过修改数据结构和添加函数来实现有向图、带权图等其他类型的图。
阅读全文
相关推荐
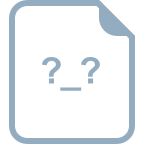




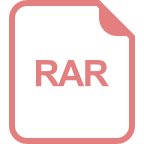
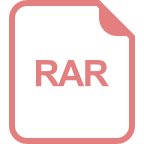
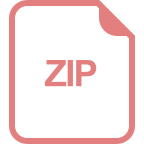
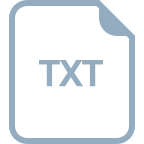
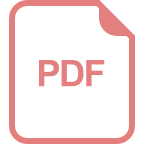
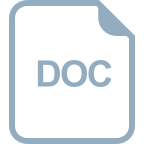



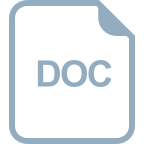

