用c语言创建一个无向图,选用邻接表法数据存储结构,并求出任意一个顶的度。同时写出图的深度,广度遍历算法
时间: 2024-02-18 08:59:40 浏览: 68
好的,我可以回答你的问题。以下是用C语言创建一个无向图的代码,使用邻接表法数据存储结构:
```
#include <stdio.h>
#include <stdlib.h>
struct Node {
int vertex;
struct Node* next;
};
struct Graph {
int numVertices;
struct Node** adjLists;
};
struct Node* createNode(int v) {
struct Node* newNode = malloc(sizeof(struct Node));
newNode->vertex = v;
newNode->next = NULL;
return newNode;
}
struct Graph* createGraph(int vertices) {
struct Graph* graph = malloc(sizeof(struct Graph));
graph->numVertices = vertices;
graph->adjLists = malloc(vertices * sizeof(struct Node*));
int i;
for (i = 0; i < vertices; i++)
graph->adjLists[i] = NULL;
return graph;
}
void addEdge(struct Graph* graph, int src, int dest) {
struct Node* newNode = createNode(dest);
newNode->next = graph->adjLists[src];
graph->adjLists[src] = newNode;
newNode = createNode(src);
newNode->next = graph->adjLists[dest];
graph->adjLists[dest] = newNode;
}
void printGraph(struct Graph* graph) {
int v;
for (v = 0; v < graph->numVertices; v++) {
struct Node* temp = graph->adjLists[v];
printf("\n Vertex %d: ", v);
while (temp) {
printf("%d -> ", temp->vertex);
temp = temp->next;
}
printf("NULL\n");
}
}
int findDegree(struct Graph* graph, int vertex) {
int degree = 0;
struct Node* temp = graph->adjLists[vertex];
while (temp) {
degree++;
temp = temp->next;
}
return degree;
}
void DFS(struct Graph* graph, int vertex, int visited[]) {
visited[vertex] = 1;
printf("%d -> ", vertex);
struct Node* temp = graph->adjLists[vertex];
while (temp) {
int adjVertex = temp->vertex;
if (!visited[adjVertex])
DFS(graph, adjVertex, visited);
temp = temp->next;
}
}
void BFS(struct Graph* graph, int startVertex) {
int visited[graph->numVertices];
int i;
for (i = 0; i < graph->numVertices; i++)
visited[i] = 0;
struct Node* queue = createNode(startVertex);
visited[startVertex] = 1;
while (queue) {
int currentVertex = queue->vertex;
printf("%d -> ", currentVertex);
struct Node* temp = graph->adjLists[currentVertex];
queue = queue->next;
while (temp) {
int adjVertex = temp->vertex;
if (!visited[adjVertex]) {
visited[adjVertex] = 1;
struct Node* newNode = createNode(adjVertex);
newNode->next = queue;
queue = newNode;
}
temp = temp->next;
}
}
}
int main() {
struct Graph* graph = createGraph(4);
addEdge(graph, 0, 1);
addEdge(graph, 0, 2);
addEdge(graph, 1, 2);
addEdge(graph, 2, 3);
printGraph(graph);
int degree = findDegree(graph, 2);
printf("\nDegree of vertex 2: %d\n", degree);
printf("\nDFS Traversal: ");
int visited[graph->numVertices];
int i;
for (i = 0; i < graph->numVertices; i++)
visited[i] = 0;
DFS(graph, 0, visited);
printf("\nBFS Traversal: ");
BFS(graph, 0);
return 0;
}
```
在这个代码中,我们首先定义了两个结构体:一个是节点(Node),用来表示图中的每个顶点,另一个是图(Graph),用来表示整个图。我们使用邻接表法来存储图,因为它比邻接矩阵法更节省空间。
我们使用createNode函数来创建一个新的节点,并使用一个指向该节点的指针来表示每个顶点的邻接表。我们使用createGraph函数来创建一个新的图,并初始化每个顶点的邻接表为空。
我们使用addEdge函数来添加边到图中,它将目标顶点添加到源顶点的邻接表中,并将源顶点添加到目标顶点的邻接表中。
我们使用printGraph函数来打印整个图,以便我们可以检查它是否正确地被创建。我们使用findDegree函数来计算任意一个顶点的度数,它遍历该顶点的邻接表并返回顶点的度数。
我们使用DFS函数和BFS函数来遍历整个图。DFS使用递归来深度优先遍历图,而BFS使用队列来广度优先遍历图。
以上是创建无向图的代码,并求出任意一个顶的度。如果您需要了解更多关于图的遍历算法的信息,请告诉我。
阅读全文
相关推荐












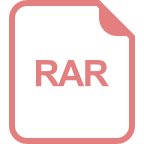





