如何在WPF中声明一个依赖属性?
时间: 2024-11-15 11:24:24 浏览: 3
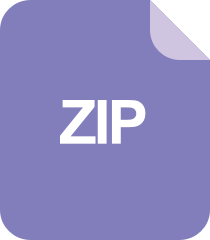
WPF入门-02依赖属性(附c#代码)
在Windows Presentation Foundation (WPF)中,声明一个依赖属性需要以下几个步骤:
1. **继承自DependencyObject**:首先,你需要创建一个新的类并从`System.Windows.DependencyObject`或其派生类继承,如`UserControl`, `Window`, 或者`DependencyObject`本身。
```csharp
public class MyCustomControl : DependencyObject
{
// ...
}
```
2. **使用`[DependencyProperty]`属性**:接下来,在类中声明依赖属性,使用`System.Windows.DependencyProperty`的静态方法`Register`。给属性起个名称,指定数据类型,还有默认值(可选)和其他属性,例如是否可写、验证规则等。
```csharp
[DependencyProperty]
public Brush BackgroundColor
{
get { return (Brush)GetValue(BackgroundColorProperty); }
set { SetValue(BackgroundColorProperty, value); }
}
// 注册属性信息,这里定义了属性名("BackgroundColor"),类型(Brush),默认值(如果有的话)
public static readonly DependencyProperty BackgroundColorProperty = DependencyProperty.Register(
"BackgroundColor", typeof(Brush), typeof(MyCustomControl),
new PropertyMetadata(null, OnBackgroundColorChanged)
);
```
- `OnBackgroundColorChanged` 是一个可选的属性更改回调,用于处理属性改变时的逻辑。
3. **验证和绑定**:你可以为属性提供验证逻辑,如果需要的话,可以通过`ValidationRules`属性添加`IDataErrorInfo`接口来实现错误检查。对于数据绑定,可以在XAML文件中直接使用`<mycontrol BackgroundColor="{Binding MyColorBrush}" />`形式。
```csharp
public object GetError(string propertyName)
{
if (value == null)
{
return string.Format("The {0} property cannot be null.", propertyName);
}
else
{
// 其他可能的验证条件...
return null;
}
}
public void SetError(string propertyName, object error)
{
_errors[propertyName] = error;
}
bool IDataErrorInfo.HasErrors => _errors.Any();
string IDataErrorInfo.Error => _errors.Aggregate((current, pair) => current + Environment.NewLine + pair.Key + ": " + pair.Value);
```
阅读全文
相关推荐
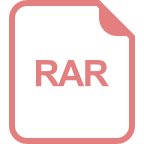
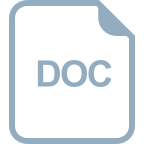
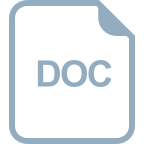
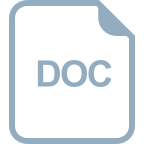
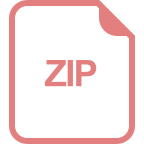
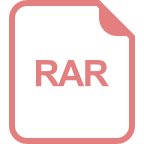
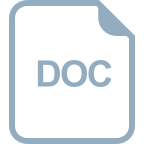
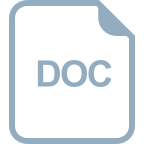
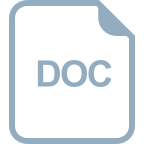
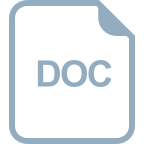
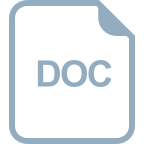
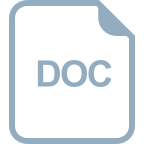
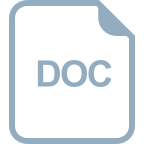
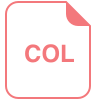


