假设nXn的稀疏矩阵A采用三元组表示,设计一个程序exp6-1.cpp实现以下功能。 (1) 生成以下两个稀疏矩阵的三元组a和b。 (2)输出a转置矩阵的三元组。(3)输出a+b的三元组。(4)输出aXb的三元组。 用c语言代码怎么写
时间: 2024-11-13 16:26:57 浏览: 35
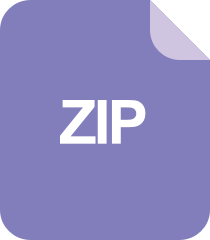
随机厄米矩阵生成器:生成一个随机的 nxn 厄米矩阵。-matlab开发
在C语言中,为了实现这个功能,我们可以利用结构体来存储稀疏矩阵的三元组(行索引、列索引和值),然后分别创建函数来完成各个操作。这里是一个简单的示例,假设我们有一个名为`sparse_matrix`的结构体以及一些辅助函数:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct sparse_triplet {
int row;
int col;
double value;
} SparseTriple;
SparseTriple* create_triplet(int r, int c, double v) {
SparseTriple *new_triplet = (SparseTriple*)malloc(sizeof(SparseTriple));
new_triplet->row = r;
new_triplet->col = c;
new_triplet->value = v;
return new_triplet;
}
void print_triplets(SparseTriple** triplets, int n, int m) {
for (int i = 0; i < n; i++) {
for (int j = 0; triplets[i][j] != NULL; j++) {
printf("(%d,%d,%lf)\t", triplets[i][j]->row, triplets[i][j]->col, triplets[i][j]->value);
}
printf("\n");
}
}
// ...其他函数用于生成矩阵、转置、加法和乘法...
// 示例:生成矩阵a和b
void generate_matrices(SparseTriple*** a, SparseTriple*** b, int n, int m, ...) {
// 实现生成a和b的代码
}
// 输出矩阵a的转置
void transpose(SparseTriple*** transposed_a, SparseTriple*** a, int n, int m) {
// 实现转置功能
}
// 输出矩阵a + b
void matrix_addition(SparseTriple*** result, SparseTriple*** a, SparseTriple*** b, int n, int m) {
// 实现矩阵加法
}
// 输出矩阵a * b
void matrix_multiplication(SparseTriple*** result, SparseTriple*** a, SparseTriple*** b, int n, int m) {
// 实现矩阵乘法,注意这里需要检查是否可以相乘
}
int main() {
int n = 5; // 假设矩阵为5x5
SparseTriple*** a = malloc(n * sizeof(SparseTriple*));
SparseTriple*** b = malloc(n * sizeof(SparseTriple*));
// 初始化矩阵a和b并生成三元组
SparseTriple*** transposed_a = malloc(n * sizeof(SparseTriple*));
transpose(transposed_a, a, n, n);
SparseTriple*** sum = malloc(n * sizeof(SparseTriple*));
matrix_addition(sum, a, b, n, n);
SparseTriple*** product = malloc(n * sizeof(SparseTriple*));
matrix_multiplication(product, a, b, n, n);
// 打印结果
print_triplets(a, n, n);
print_triplets(transposed_a, n, n);
print_triplets(sum, n, n);
print_triplets(product, n, n);
free(*a);
free(*b);
free(transposed_a);
free(sum);
free(product);
return 0;
}
```
请注意,这只是一个基本的框架,实际实现还需要处理更多细节,比如内存分配、输入验证等。同时,矩阵乘法部分更复杂,因为需要考虑稀疏矩阵乘法规则(通常涉及到邻接计算)。此外,上述代码未提供生成矩阵的具体函数,你需要自定义这部分。
阅读全文
相关推荐
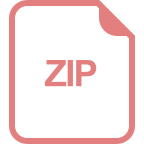
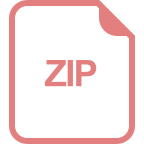
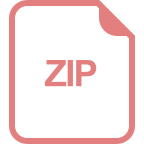
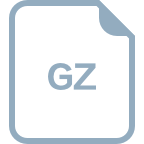
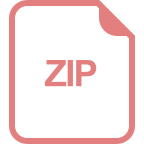
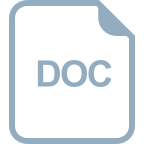
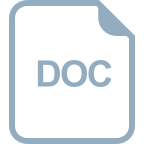
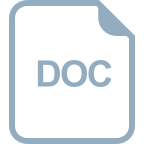








