疲劳驾驶检测python
时间: 2023-07-28 19:09:22 浏览: 172
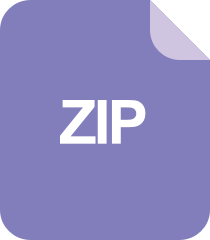
基于 python实现的双端计算的疲劳驾驶检测
对于疲劳驾驶检测,你可以使用Python来进行相关的开发。以下是一个简单的示例代码,用于检测驾驶员的疲劳程度:
```python
import cv2
import dlib
from scipy.spatial import distance
def calculate_eye_aspect_ratio(eye):
# 计算眼睛纵横比
A = distance.euclidean(eye[1], eye[5])
B = distance.euclidean(eye[2], eye[4])
C = distance.euclidean(eye[0], eye[3])
ear = (A + B) / (2.0 * C)
return ear
def detect_fatigue(frame, detector, predictor, ear_threshold):
# 检测疲劳驾驶
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
faces = detector(gray)
for face in faces:
landmarks = predictor(gray, face)
left_eye = []
right_eye = []
for n in range(36, 42):
x = landmarks.part(n).x
y = landmarks.part(n).y
left_eye.append((x, y))
for n in range(42, 48):
x = landmarks.part(n).x
y = landmarks.part(n).y
right_eye.append((x, y))
left_ear = calculate_eye_aspect_ratio(left_eye)
right_ear = calculate_eye_aspect_ratio(right_eye)
avg_ear = (left_ear + right_ear) / 2
if avg_ear < ear_threshold:
return True
return False
# 加载人脸检测器和人脸特征点检测器
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat")
# 打开摄像头
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
if not ret:
break
# 设置疲劳阈值,根据实际情况调整
ear_threshold = 0.25
if detect_fatigue(frame, detector, predictor, ear_threshold):
cv2.putText(frame, "Fatigue Detected", (10, 30), cv2.FONT_HERSHEY_SIMPLEX, 0.7, (0, 0, 255), 2)
cv2.imshow("Frame", frame)
if cv2.waitKey(1) == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
```
这段代码使用了dlib库进行人脸检测和人脸特征点检测,并计算了眼睛纵横比(eye aspect ratio, EAR)来判断驾驶员是否疲劳。你需要下载dlib库和shape_predictor_68_face_landmarks.dat文件,并将其放在代码同一目录下。
代码中通过摄像头实时获取图像,然后检测人脸并计算眼睛纵横比。如果眼睛纵横比小于设定的阈值,将会显示"Fatigue Detected"的提示。
请注意,这只是一个简单的示例代码,实际应用中可能需要更复杂的算法和模型来进行疲劳驾驶检测。
阅读全文
相关推荐
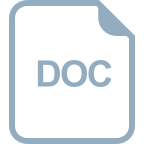
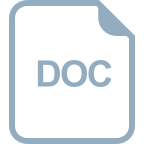

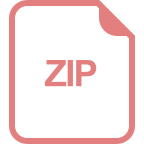
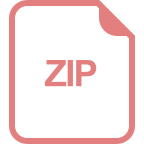


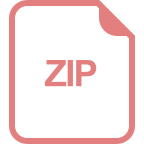
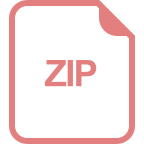
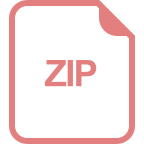
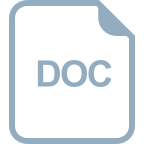
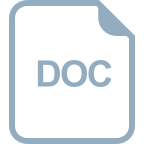
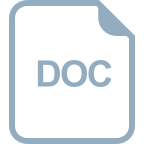
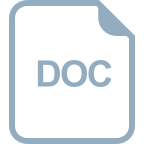



