帮我用JAVA写一段netty客户端代码的demo
时间: 2024-09-29 14:12:53 浏览: 41
好的,以下是使用Netty Java编写的简单TCP客户端示例:
```java
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
public class NettyClient {
private static final String SERVER_HOST = "localhost";
private static final int SERVER_PORT = 8080; // 替换为你服务器的实际端口
public static void main(String[] args) throws Exception {
EventLoopGroup group = new NioEventLoopGroup(); // 使用NIO线程池
try {
Bootstrap bootstrap = new Bootstrap();
bootstrap.group(group)
.channel(NioSocketChannel.class) // 设置通道类型
.handler(new ClientHandler()); // 客户端处理器
ChannelFuture channelFuture = bootstrap.connect(SERVER_HOST, SERVER_PORT).sync(); // 连接服务器
System.out.println("Connected to server at " + channelFuture.channel().remoteAddress());
// 读取数据并处理
byte[] buffer = new byte[1024];
while (true) {
int length = channelFuture.channel().read(buffer);
if (length == -1) break; // 如果读取结束则退出循环
String receivedData = new String(buffer, 0, length); // 转换字节数组为字符串
System.out.println("Received: " + receivedData);
// 这里可以添加你的业务处理逻辑
}
} finally {
group.shutdownGracefully(); // 关闭线程池
}
}
static class ClientHandler extends ChannelInitializer<SocketChannel> {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new StringDecoder()); // 解码字节到字符串
ch.pipeline().addLast(new SimpleClientInboundHandler<String>() { // 自定义接收消息处理器
@Override
public void channelRead0(ChannelHandlerContext ctx, String msg) {
System.out.println("Received from server: " + msg);
// 在这里发送回复或者其他操作
}
});
}
}
}
// 相关问题:
1. 如何在Netty中处理异常?
2. 这段代码是否支持SSL加密通信?如果需要怎么做?
3. 如何在Netty中实现断点续传功能? >>
相关推荐
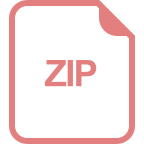
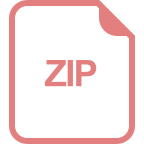
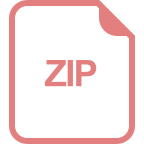














