如何用C语言实现一个基本的单向链表数据结构?
时间: 2024-10-29 16:16:32 浏览: 4
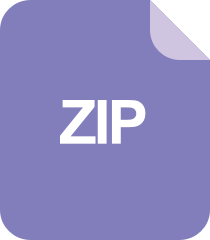
C语言实现单向链表及操作

在C语言中,我们可以使用结构体(struct)和指针来实现一个简单的单向链表。以下是创建、插入和删除节点的基本步骤:
1. 定义链表节点结构(Node):
```c
typedef struct Node {
int data; // 节点存储的数据
struct Node* next; // 指向下一个节点的指针
} Node;
```
2. 创建链表头结点(head)并初始化为NULL:
```c
Node* head = NULL;
```
3. 插入节点:
- 插入到链表头部:
```c
void insertAtFront(int value) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 分配内存
newNode->data = value;
newNode->next = head;
head = newNode;
}
```
- 插入到链表尾部:
```c
void insertAtEnd(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
if (head == NULL) {
head = newNode; // 链表为空,直接插入
} else {
Node* temp = head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
```
4. 删除节点:
- 删除特定值的节点:
```c
void deleteNodeWithValue(int value) {
Node* temp = head;
Node* prev = NULL;
if (temp != NULL && temp->data == value) {
head = temp->next;
free(temp); // 释放内存
return;
}
while (temp != NULL && temp->data != value) {
prev = temp;
temp = temp->next;
}
if (temp == NULL) return; // 找不到该值
prev->next = temp->next;
free(temp);
}
```
5. 遍历链表:
```c
void printList() {
Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
```
阅读全文
相关推荐
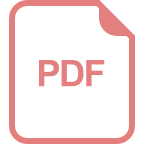
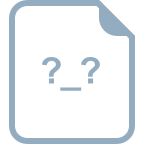
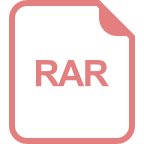
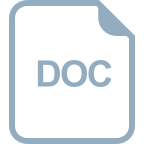
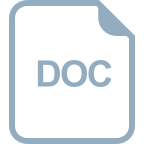
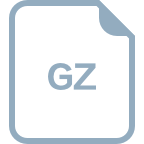
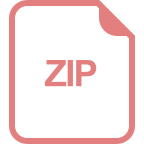
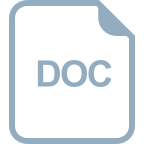
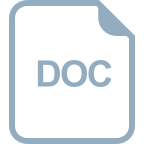
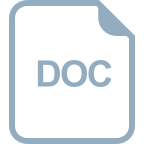
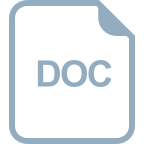
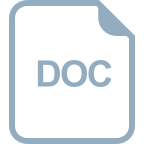
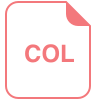


