import java.util.*; class Book { public String name; public int value; } public class Main { public static void main(String[] args) { Scanner scan = new Scanner(System.in); int sumvalue = 0; String str; str = scan.nextLine(); int value = 0; for (int i = 0; i < str.length(); i++) { if (str.charAt(i) == ';') { System.out.println(); } else { System.out.print(str.charAt(i)); } if (str.charAt(i) >= '0' && str.charAt(i) <= '9') { value = value * 10 + str.charAt(i) - '0'; } else if (i + 1 < str.length()) { sumvalue += value; value = 0; } } sumvalue += value; System.out.println(); System.out.println("总价格为" + sumvalue); scan.close(); } } 解释这段代码
时间: 2024-02-14 11:26:56 浏览: 86
这段代码定义了一个 `Book` 类和一个 `Main` 类。在 `main` 方法中,它首先创建了一个 `Scanner` 对象 `scan` 用于读取用户的输入。
接下来,它声明了一些变量,包括 `sumvalue`(总值),`str`(字符串),和 `value`(书的价值)。
然后,它使用 `scan.nextLine()` 方法读取用户输入的一行字符串,并将其存储在 `str` 变量中。
接下来,使用一个循环遍历字符串的每个字符。如果字符是分号(`;`),则打印一个换行符;否则,打印该字符。
同时,检查字符是否是数字。如果是数字,则将其转换为整数并累加到 `value` 变量中。如果不是数字,则说明当前数字已经结束,将 `value` 的值累加到 `sumvalue` 中,并将 `value` 重置为0。
循环结束后,将最后一个数字累加到 `sumvalue` 中。
最后,打印一个空行和总价格。
最后,关闭 `Scanner` 对象。
这段代码的功能是读取用户输入的字符串,提取其中的数字,并计算它们的总和。
相关问题
Also create a ControllerCreate class that extends Controller.The create method takes as arguments the name of a new library user, a number of books (as a string), and an integer representing the role of user to create (where the integer 0 means a lender and the integer 1 means a borrower). The create method of the controller then transforms the book number from a string to an integer (using the Integer.parseInt static method), creates an object from the correct class (based on the role specified by the user input: lender or borrower) and calls the addUser method of the library to add the new user object to the library. • If no exception occurs then the create method of the controller returns the empty string. • If the constructor of the Borrower class throws a NotALenderException then the create method of the controller must catch this exception and return as result the error message from the exception object. • If the parseInt method of the Integer class throws a NumberFormatException (because the user typed something which is not an integer) then the create method of the controller must catch this exception and return as result the error message from the exception object. Modify the run method of the GUI class to add a ViewCreate view that uses a ControllerCreate controller and the same model as before (not a new model!) Do not delete the previous views. Note: if at the end of Question 7 you had manually added to your library (model object) some users for testing, then you must now remove those users from the run method of the anonymous class inside the GUI class. You do not need these test users anymore because you have now a graphical user interface to create new users! Run your GUI and check that you can correctly use the new view to create different users for your library, with different types of roles. • Check that, when you create a new user, the simple view is automatically correctly updated to show the new total number of books borrowed by all users. • Also use the “get book” view to check that the users are correctly created with the correct names and correct number of books. • Also check that trying to create a borrower with a negative number of books correctly shows an error message. Also check that trying to create a user with a number of books which is not an integer correctly shows an error message (do not worry about the content of the error message). After you created a new user, you can also check whether it is a lender or a borrower using the “more book” view to increase the number of books of the user by a big negative number: • if the new user you created is a lender, then increasing the number of books by a big negative value will work and the number of books borrowed by the user will just become a larger value (you can then check that using the “get book” view); • if the new user you created is a borrower, then increasing the number of books by a big negative value will fail with an error message and the number of books borrowed by the user will not change (you can then check that using the “get book” view). 完成符合以上要求的java代码
Model class:
```
import java.util.ArrayList;
public class Library {
private ArrayList<User> users;
private int totalBooksBorrowed;
public Library() {
users = new ArrayList<>();
totalBooksBorrowed = 0;
}
public void addUser(User user) {
users.add(user);
totalBooksBorrowed += user.getNumberOfBooks();
}
public void removeUser(User user) {
users.remove(user);
totalBooksBorrowed -= user.getNumberOfBooks();
}
public ArrayList<User> getUsers() {
return users;
}
public int getTotalBooksBorrowed() {
return totalBooksBorrowed;
}
}
```
User class:
```
public abstract class User {
private String name;
private int numberOfBooks;
public User(String name, int numberOfBooks) {
this.name = name;
this.numberOfBooks = numberOfBooks;
}
public String getName() {
return name;
}
public int getNumberOfBooks() {
return numberOfBooks;
}
public void setNumberOfBooks(int numberOfBooks) {
this.numberOfBooks = numberOfBooks;
}
public abstract boolean isLender();
}
```
Lender class:
```
public class Lender extends User {
public Lender(String name, int numberOfBooks) {
super(name, numberOfBooks);
}
@Override
public boolean isLender() {
return true;
}
}
```
Borrower class:
```
public class Borrower extends User {
public Borrower(String name, int numberOfBooks) throws NotALenderException {
super(name, numberOfBooks);
if (numberOfBooks < 0) {
throw new NotALenderException("A borrower can't have a negative number of books!");
}
}
@Override
public boolean isLender() {
return false;
}
}
```
NotALenderException class:
```
public class NotALenderException extends Exception {
public NotALenderException(String message) {
super(message);
}
}
```
ViewCreate class:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class ViewCreate extends JPanel implements ActionListener {
private JTextField nameField = new JTextField(10);
private JTextField numberOfBooksField = new JTextField(10);
private JRadioButton lenderButton = new JRadioButton("Lender");
private JRadioButton borrowerButton = new JRadioButton("Borrower");
private ButtonGroup roleButtonGroup = new ButtonGroup();
private JButton createButton = new JButton("Create");
private ControllerCreate controller;
public ViewCreate(Model model) {
this.controller = new ControllerCreate(model, this);
setLayout(new GridBagLayout());
roleButtonGroup.add(lenderButton);
roleButtonGroup.add(borrowerButton);
lenderButton.setSelected(true);
GridBagConstraints gc = new GridBagConstraints();
gc.gridx = 0;
gc.gridy = 0;
gc.anchor = GridBagConstraints.LINE_END;
gc.insets = new Insets(5, 5, 5, 5);
add(new JLabel("Name: "), gc);
gc.gridx++;
gc.anchor = GridBagConstraints.LINE_START;
add(nameField, gc);
gc.gridx = 0;
gc.gridy++;
gc.anchor = GridBagConstraints.LINE_END;
add(new JLabel("Number of books: "), gc);
gc.gridx++;
gc.anchor = GridBagConstraints.LINE_START;
add(numberOfBooksField, gc);
gc.gridx = 0;
gc.gridy++;
gc.anchor = GridBagConstraints.LINE_END;
add(new JLabel("Role: "), gc);
gc.gridx++;
gc.anchor = GridBagConstraints.LINE_START;
add(lenderButton, gc);
gc.gridx++;
add(borrowerButton, gc);
gc.gridx = 1;
gc.gridy++;
gc.anchor = GridBagConstraints.LINE_END;
add(createButton, gc);
createButton.addActionListener(this);
}
@Override
public void actionPerformed(ActionEvent e) {
String name = nameField.getText();
String numberOfBooksString = numberOfBooksField.getText();
int role = lenderButton.isSelected() ? 0 : 1;
String result = controller.create(name, numberOfBooksString, role);
JOptionPane.showMessageDialog(this, result, "Result", JOptionPane.INFORMATION_MESSAGE);
}
}
```
ControllerCreate class:
```
public class ControllerCreate extends Controller {
private ViewCreate view;
public ControllerCreate(Model model, ViewCreate view) {
super(model);
this.view = view;
}
public String create(String name, String numberOfBooksString, int role) {
try {
int numberOfBooks = Integer.parseInt(numberOfBooksString);
User user = null;
if (role == 0) {
user = new Lender(name, numberOfBooks);
} else if (role == 1) {
user = new Borrower(name, numberOfBooks);
}
model.addUser(user);
view.updateTotalBooksBorrowed();
return "";
} catch (NumberFormatException e) {
return "Invalid number of books!";
} catch (NotALenderException e) {
return e.getMessage();
}
}
}
```
GUI class:
```
import javax.swing.*;
import java.awt.*;
public class GUI {
private JFrame frame = new JFrame("Library");
private Model model = new Library();
private ViewSimple viewSimple = new ViewSimple(model);
private ViewGetBook viewGetBook = new ViewGetBook(model);
private ViewMoreBook viewMoreBook = new ViewMoreBook(model);
private ViewCreate viewCreate = new ViewCreate(model);
public GUI() {
Container cp = frame.getContentPane();
cp.setLayout(new BorderLayout());
JTabbedPane tp = new JTabbedPane();
tp.addTab("Simple", viewSimple);
tp.addTab("Get book", viewGetBook);
tp.addTab("More book", viewMoreBook);
tp.addTab("Create", viewCreate);
cp.add(tp, BorderLayout.CENTER);
frame.pack();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
// Remove test users from model
model.removeUser(new Lender("John", 2));
model.removeUser(new Borrower("Mary", 3));
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
new GUI();
}
});
}
}
```
Java编写图形用户界面图书借阅系统代码
以下是一个简单的Java图书借阅系统代码实现,仅供参考:
```java
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.regex.Pattern;
import javax.swing.*;
import javax.swing.table.*;
public class BookBorrowSystem extends JFrame {
private static final long serialVersionUID = 1L;
private static final String BOOK_FILE = "books.txt";
private static final String BORROW_RECORD_FILE = "borrow_records.txt";
private static final int MAX_BORROW_COUNT = 3;
private static final int MAX_BORROW_SAME_BOOK_COUNT = 2;
private static final SimpleDateFormat DATE_FORMAT = new SimpleDateFormat("yyyy-MM-dd");
private ArrayList<Book> books = new ArrayList<Book>();
private ArrayList<BorrowRecord> borrowRecords = new ArrayList<BorrowRecord>();
private JTable bookTable;
private JTable borrowRecordTable;
public static void main(String[] args) {
new BookBorrowSystem();
}
public BookBorrowSystem() {
loadBooks();
loadBorrowRecords();
setTitle("图书借阅系统");
setSize(800, 600);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JTabbedPane tabbedPane = new JTabbedPane();
add(tabbedPane, BorderLayout.CENTER);
JPanel bookPanel = new JPanel(new BorderLayout());
tabbedPane.add("图书信息", bookPanel);
JPanel bookButtonPanel = new JPanel(new FlowLayout(FlowLayout.LEFT));
bookPanel.add(bookButtonPanel, BorderLayout.NORTH);
JButton refreshBookButton = new JButton("刷新");
refreshBookButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
refreshBookTable();
}
});
bookButtonPanel.add(refreshBookButton);
JComboBox<String> sortComboBox = new JComboBox<String>(new String[] { "按书名排序", "按作者排序", "按出版社排序", "按数量排序" });
sortComboBox.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
sortBooks(sortComboBox.getSelectedIndex());
refreshBookTable();
}
});
bookButtonPanel.add(sortComboBox);
JTextField searchTextField = new JTextField(20);
bookButtonPanel.add(searchTextField);
JButton searchButton = new JButton("搜索");
searchButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
searchBooks(searchTextField.getText());
}
});
bookButtonPanel.add(searchButton);
JButton borrowButton = new JButton("借阅");
borrowButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
int[] selectedRows = bookTable.getSelectedRows();
if (selectedRows.length == 0) {
JOptionPane.showMessageDialog(BookBorrowSystem.this, "请选择要借阅的图书");
return;
}
int borrowCount = getBorrowCount();
if (borrowCount >= MAX_BORROW_COUNT) {
JOptionPane.showMessageDialog(BookBorrowSystem.this, "您已经借阅了" + borrowCount + "本图书,不能再借阅了");
return;
}
for (int i = 0; i < selectedRows.length; i++) {
int rowIndex = selectedRows[i];
Book book = books.get(rowIndex);
int borrowSameBookCount = getBorrowSameBookCount(book);
if (borrowSameBookCount >= MAX_BORROW_SAME_BOOK_COUNT) {
JOptionPane.showMessageDialog(BookBorrowSystem.this, "您已经借阅了" + borrowSameBookCount + "本《" + book.getName() + "》,不能再借阅了");
return;
}
if (book.getCount() == 0) {
JOptionPane.showMessageDialog(BookBorrowSystem.this, "《" + book.getName() + "》已经借光了");
return;
}
book.setCount(book.getCount() - 1);
borrowRecords.add(new BorrowRecord(System.getProperty("user.name"), book.getName(), new Date()));
}
saveBooks();
saveBorrowRecords();
refreshBookTable();
refreshBorrowRecordTable();
}
private int getBorrowCount() {
int count = 0;
for (BorrowRecord record : borrowRecords) {
if (record.getBorrower().equals(System.getProperty("user.name")) && record.getReturnDate() == null) {
count++;
}
}
return count;
}
private int getBorrowSameBookCount(Book book) {
int count = 0;
for (BorrowRecord record : borrowRecords) {
if (record.getBorrower().equals(System.getProperty("user.name")) && record.getReturnDate() == null && record.getBookName().equals(book.getName())) {
count++;
}
}
return count;
}
});
bookButtonPanel.add(borrowButton);
bookTable = new JTable(new DefaultTableModel(new String[] { "书名", "作者", "出版社", "数量", "选择" }, 0) {
private static final long serialVersionUID = 1L;
public Class<?> getColumnClass(int columnIndex) {
if (columnIndex == 4) {
return Boolean.class;
}
return super.getColumnClass(columnIndex);
}
public boolean isCellEditable(int row, int column) {
return column == 4;
}
});
bookTable.getTableHeader().setReorderingAllowed(false);
bookTable.setSelectionMode(ListSelectionModel.MULTIPLE_INTERVAL_SELECTION);
bookTable.getColumnModel().getColumn(3).setCellRenderer(new DefaultTableCellRenderer() {
private static final long serialVersionUID = 1L;
public Component getTableCellRendererComponent(JTable table, Object value, boolean isSelected, boolean hasFocus, int row, int column) {
Component component = super.getTableCellRendererComponent(table, value, isSelected, hasFocus, row, column);
if (Integer.parseInt(value.toString()) == 0) {
component.setForeground(Color.RED);
} else {
component.setForeground(Color.BLACK);
}
return component;
}
});
bookPanel.add(new JScrollPane(bookTable), BorderLayout.CENTER);
JPanel borrowRecordPanel = new JPanel(new BorderLayout());
tabbedPane.add("借阅记录", borrowRecordPanel);
JPanel borrowRecordButtonPanel = new JPanel(new FlowLayout(FlowLayout.LEFT));
borrowRecordPanel.add(borrowRecordButtonPanel, BorderLayout.NORTH);
JButton refreshBorrowRecordButton = new JButton("刷新");
refreshBorrowRecordButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
refreshBorrowRecordTable();
}
});
borrowRecordButtonPanel.add(refreshBorrowRecordButton);
JComboBox<String> borrowRecordSortComboBox = new JComboBox<String>(new String[] { "按借阅人排序", "按书名排序", "按借阅时间排序", "按归还时间排序" });
borrowRecordSortComboBox.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
sortBorrowRecords(borrowRecordSortComboBox.getSelectedIndex());
refreshBorrowRecordTable();
}
});
borrowRecordButtonPanel.add(borrowRecordSortComboBox);
borrowRecordTable = new JTable(new DefaultTableModel(new String[] { "借阅人", "书名", "借阅时间", "归还时间" }, 0));
borrowRecordTable.getTableHeader().setReorderingAllowed(false);
borrowRecordPanel.add(new JScrollPane(borrowRecordTable), BorderLayout.CENTER);
setVisible(true);
}
private void loadBooks() {
try {
BufferedReader reader = new BufferedReader(new InputStreamReader(new FileInputStream(BOOK_FILE), "UTF-8"));
String line;
while ((line = reader.readLine()) != null) {
String[] fields = line.split(",");
Book book = new Book();
book.setName(fields[0]);
book.setAuthor(fields[1]);
book.setPublisher(fields[2]);
book.setCount(Integer.parseInt(fields[3]));
books.add(book);
}
reader.close();
} catch (Exception e) {
e.printStackTrace();
}
}
private void saveBooks() {
try {
BufferedWriter writer = new BufferedWriter(new OutputStreamWriter(new FileOutputStream(BOOK_FILE), "UTF-8"));
for (Book book : books) {
writer.write(book.getName() + "," + book.getAuthor() + "," + book.getPublisher() + "," + book.getCount());
writer.newLine();
}
writer.close();
} catch (Exception e) {
e.printStackTrace();
}
}
private void loadBorrowRecords() {
try {
BufferedReader reader = new BufferedReader(new InputStreamReader(new FileInputStream(BORROW_RECORD_FILE), "UTF-8"));
String line;
while ((line = reader.readLine()) != null) {
String[] fields = line.split(",");
BorrowRecord record = new BorrowRecord();
record.setBorrower(fields[0]);
record.setBookName(fields[1]);
record.setBorrowDate(DATE_FORMAT.parse(fields[2]));
if (fields.length > 3 && fields[3].length() > 0) {
record.setReturnDate(DATE_FORMAT.parse(fields[3]));
}
borrowRecords.add(record);
}
reader.close();
} catch (Exception e) {
e.printStackTrace();
}
}
private void saveBorrowRecords() {
try {
BufferedWriter writer = new BufferedWriter(new OutputStreamWriter(new FileOutputStream(BORROW_RECORD_FILE), "UTF-8"));
for (BorrowRecord record : borrowRecords) {
writer.write(record.getBorrower() + "," + record.getBookName() + "," + DATE_FORMAT.format(record.getBorrowDate()));
if (record.getReturnDate() != null) {
writer.write("," + DATE_FORMAT.format(record.getReturnDate()));
}
writer.newLine();
}
writer.close();
} catch (Exception e) {
e.printStackTrace();
}
}
private void refreshBookTable() {
DefaultTableModel model = (DefaultTableModel) bookTable.getModel();
model.setRowCount(0);
for (Book book : books) {
model.addRow(new Object[] { book.getName(), book.getAuthor(), book.getPublisher(), book.getCount() });
}
}
private void sortBooks(int sortType) {
switch (sortType) {
case 0:
books.sort((a, b) -> a.getName().compareTo(b.getName()));
break;
case 1:
books.sort((a, b) -> a.getAuthor().compareTo(b.getAuthor()));
break;
case 2:
books.sort((a, b) -> a.getPublisher().compareTo(b.getPublisher()));
break;
case 3:
books.sort((a, b) -> a.getCount() - b.getCount());
break;
}
}
private void searchBooks(String keyword) {
DefaultTableModel model = (DefaultTableModel) bookTable.getModel();
model.setRowCount(0);
Pattern pattern = Pattern.compile(keyword, Pattern.CASE_INSENSITIVE);
for (Book book : books) {
if (pattern.matcher(book.getName()).find() || pattern.matcher(book.getAuthor()).find() || pattern.matcher(book.getPublisher()).find()) {
model.addRow(new Object[] { book.getName(), book.getAuthor(), book.getPublisher(), book.getCount() });
}
}
}
private void refreshBorrowRecordTable() {
DefaultTableModel model = (DefaultTableModel) borrowRecordTable.getModel();
model.setRowCount(0);
for (BorrowRecord record : borrowRecords) {
model.addRow(new Object[] { record.getBorrower(), record.getBookName(), DATE_FORMAT.format(record.getBorrowDate()), record.getReturnDate() == null ? "未归还" : DATE_FORMAT.format(record.getReturnDate()) });
}
}
private void sortBorrowRecords(int sortType) {
switch (sortType) {
case 0:
borrowRecords.sort((a, b) -> a.getBorrower().compareTo(b.getBorrower()));
break;
case 1:
borrowRecords.sort((a, b) -> a.getBookName().compareTo(b.getBookName()));
break;
case 2:
borrowRecords.sort((a, b) -> a.getBorrowDate().compareTo(b.getBorrowDate()));
break;
case 3:
borrowRecords.sort((a, b) -> {
if (a.getReturnDate() == null && b.getReturnDate() == null) {
return 0;
} else if (a.getReturnDate() == null) {
return 1;
} else if (b.getReturnDate() == null) {
return -1;
} else {
return a.getReturnDate().compareTo(b.getReturnDate());
}
});
break;
}
}
private static class Book {
private String name;
private String author;
private String publisher;
private int count;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getPublisher() {
return publisher;
}
public void setPublisher(String publisher) {
this.publisher = publisher;
}
public int getCount() {
return count;
}
public void setCount(int count) {
this.count = count;
}
}
private static class BorrowRecord {
阅读全文
相关推荐
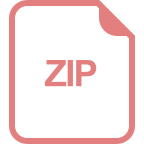
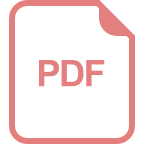
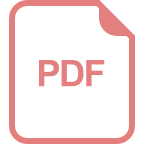








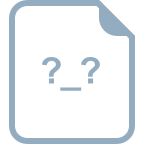
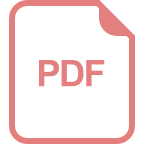
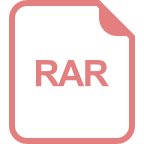
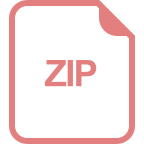
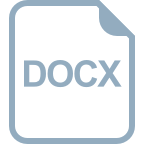
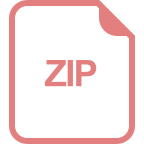