C语言学生成绩管理系统链表
时间: 2024-12-20 17:10:20 浏览: 4
在C语言中,设计一个成绩管理系统链表通常涉及到以下几个步骤:
1. 定义数据结构:首先,我们需要创建一个节点结构体,包含学生的ID、姓名和成绩等属性。例如:
```c
typedef struct {
int student_id;
char name[50];
float score;
} StudentNode;
```
2. 链表定义:定义一个链表结构,包括头指针和节点的数据结构。
```c
typedef struct {
StudentNode* head;
int length;
} ScoreList;
```
3. 创建函数:编写插入节点、删除节点、查找节点、添加成绩等功能的函数。比如插入节点可以这样实现:
```c
void insert(StudentNode** head, int id, char* name, float score) {
StudentNode* newNode = (StudentNode*)malloc(sizeof(StudentNode));
newNode->student_id = id;
strcpy(newNode->name, name);
newNode->score = score;
newNode->next = *head;
*head = newNode;
}
```
4. 查找成绩:遍历链表找到对应学生并获取其成绩。
```c
float findScore(ScoreList* list, int id) {
StudentNode* current = list->head;
while (current != NULL && current->student_id != id) {
current = current->next;
}
if (current != NULL) return current->score;
else return -1; // 表示未找到
}
```
5. 删除节点:根据需要提供删除节点的功能。
阅读全文
相关推荐
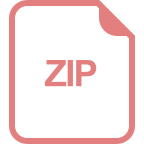
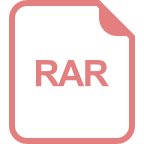















