pid esp32 Arduino示例代码
时间: 2024-11-23 09:28:31 浏览: 72
PID (Proportional-Integral-Derivative)控制器是一种用于自动控制系统中,根据当前状态、偏差以及变化率来实时调整的算法。在ESP32(Espressif Systems的ESP32系列微控制器)和Arduino平台上,可以编写PID控制器的示例代码来调节电机速度、温度或其他线性或非线性系统的性能。这里是一个基础的PID控制代码片段:
```cpp
#include <PID_v1.h>
// ESP32硬件配置
const int setpointPin = D2; // 设定点输入
const int inputPin = D0; // 测量反馈输入
const int outputPin = D3; // 输出给电机的信号
// PID设置
PID myPID(PID::Mode PID_MODE, float Kp, float Ki, float Kd);
float lastError = 0;
float integralAccumulator = 0;
void setup() {
Serial.begin(9600);
// 初始化PID对象
myPID.setSampleTime(0.01); // 更新周期,单位秒
myPID.SetKp(Kp); // 比例系数
myPID.SetKi(Ki); // 积分系数
myPID.SetKd(Kd); // 微分系数
}
void loop() {
// 获取设定点和反馈值
float desiredValue = analogRead(setpointPin);
float measuredValue = analogRead(inputPin);
// 计算误差
float error = desiredValue - measuredValue;
// 计算积分项
integralAccumulator += error * myPID.getSampleTime();
// 防止积分爆炸,限制积分范围
if (integralAccumulator > PID_MAX_INTEGRAL) {
integralAccumulator = PID_MAX_INTEGRAL;
} else if (integralAccumulator < -PID_MAX_INTEGRAL) {
integralAccumulator = -PID_MAX_INTEGRAL;
}
// 计算PID输出
float output = myPID.Compute(error, integralAccumulator, getDerivative());
// 发送PWM信号给电机
analogWrite(outputPin, map(output, -PID_OUTPUT_RANGE, PID_OUTPUT_RANGE, 0, 255));
// 打印日志
Serial.print("Error: ");
Serial.println(error);
Serial.print("Output: ");
Serial.println(output);
delay(100);
}
float getDerivative() {
// 如果需要计算微分项,可以用时间差和两次测量值计算速度
// 这里仅示例,未实际实现,因为ESP32不是专业的计时器,可能存在精度问题
return 0.0;
}
```
请根据实际硬件需求调整参数,如比例系数(Kp)、积分系数(Ki)和微分系数(Kd),并确保有足够的硬件支持和足够稳定的电源。在使用过程中,你可能还需要针对具体的系统进行调试和PID参数的优化。
阅读全文
相关推荐
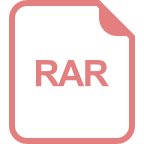
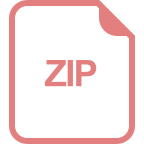
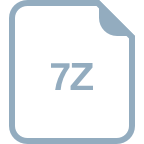




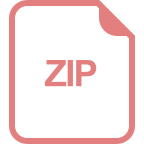
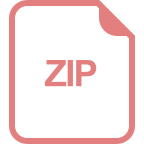
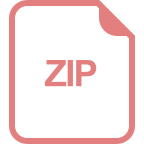
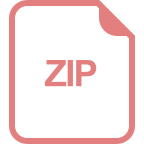
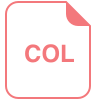
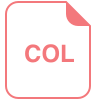
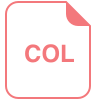




