飞机大战面向对象c++
时间: 2024-07-15 14:00:59 浏览: 74
"飞机大战"通常是一个经典的计算机游戏,用面向对象的 C++ 开发可以更好地组织代码结构和复用性。以下是一个简单的面向对象设计思路:
1. **类设计**:
- **飞机类**: 包含属性(如位置、速度、生命值)、方法(移动、射击、碰撞检测)。
- **子弹类**: 子弹的简单表示,包含发射位置、速度、生命周期等属性,以及更新和销毁的方法。
- **敌机类**: 类似飞机,但可能有不同的行为模式和生命值。
2. **继承与多态**:
- **子类继承**: 飞机类可能被敌机和玩家飞机继承,这样可以重用部分代码,每个子类可以根据需要覆盖或添加特定行为。
- **接口/抽象基类**: 如果多个子类共享一些行为,可以创建一个抽象基类,让它们实现。
3. **事件驱动**:
- 使用事件循环处理用户输入(键盘事件)、定时器(更新飞机位置)和碰撞检测事件。
4. **资源管理**:
- 使用智能指针(如 shared_ptr 或 unique_ptr)来管理游戏中的资源,如图片、声音等。
5. **游戏主循环**:
- 游戏主循环负责调度各个对象的执行,更新游戏状态并渲染画面。
相关问题
C++面向对象实现飞机大战
好的,我会尽力回答你的问题。
首先,飞机大战是一款经典的游戏,需要使用C++面向对象的编程思想来实现。
在实现飞机大战时,我们可以将游戏中的各个元素都抽象成对象,例如玩家飞机、敌机、子弹、道具等。我们可以使用类来表示这些对象,并定义它们的属性和方法。
下面是一个示例代码:
```c++
class Plane {
public:
int x, y; // 飞机坐标
int speed; // 飞机速度
int health; // 飞机生命值
void moveUp() { y -= speed; }
void moveDown() { y += speed; }
void moveLeft() { x -= speed; }
void moveRight() { x += speed; }
void fire() { /* 发射子弹 */ }
};
class Bullet {
public:
int x, y; // 子弹坐标
int speed; // 子弹速度
int damage; // 子弹伤害
void move() { y -= speed; }
};
class Enemy {
public:
int x, y; // 敌机坐标
int speed; // 敌机速度
int health; // 敌机生命值
void move() { y += speed; }
};
class Prop {
public:
int x, y; // 道具坐标
int speed; // 道具速度
void move() { y += speed; }
};
// 游戏主函数
int main() {
Plane playerPlane;
Bullet bullets[100];
Enemy enemies[100];
Prop props[100];
// 游戏逻辑代码
// ...
}
```
在这个示例代码中,我们定义了四个类:`Plane`、`Bullet`、`Enemy`、`Prop`,分别表示玩家飞机、子弹、敌机和道具。每个类都有自己的属性和方法,用来描述该对象的状态和行为。
在游戏主函数中,我们创建了若干个对象,用来表示游戏中的各个元素。通过调用对象的方法,我们可以实现对象的移动、攻击等行为。
当然,这只是一个简单的示例代码,实际上,实现一个完整的飞机大战游戏需要更多的代码和逻辑。但是,通过面向对象的编程思想,我们可以更好地组织代码,提高代码的复用性和可维护性。
用c++面向对象的方式完成飞机大战
好的,我很乐意回答你的问题。以下是使用C++面向对象的方式完成飞机大战的基本步骤:
1. 首先,需要定义一个基类来表示游戏中的物体,例如飞机、子弹和敌人。
```c++
class GameObject {
protected:
int x, y; // 物体的坐标
int speed; // 物体的速度
int hp; // 物体的生命值
public:
virtual void move() = 0; // 纯虚函数,表示物体的移动
virtual void draw() = 0; // 纯虚函数,表示物体的绘制
};
```
2. 接下来,定义飞机、子弹和敌人类,它们都是GameObject的子类。
```c++
class Plane : public GameObject {
private:
int score; // 飞机的得分
public:
void move(); // 实现抽象函数,表示飞机的移动
void draw(); // 实现抽象函数,表示飞机的绘制
void shoot(); // 飞机发射子弹
};
class Bullet : public GameObject {
public:
void move(); // 实现抽象函数,表示子弹的移动
void draw(); // 实现抽象函数,表示子弹的绘制
};
class Enemy : public GameObject {
public:
void move(); // 实现抽象函数,表示敌人的移动
void draw(); // 实现抽象函数,表示敌人的绘制
};
```
3. 定义游戏类,它包含游戏中所有的对象,负责游戏逻辑的处理和绘制。
```c++
class Game {
private:
Plane plane; // 玩家飞机
vector<Bullet> bullets; // 所有子弹
vector<Enemy> enemies; // 所有敌人
int score; // 玩家得分
public:
void init(); // 初始化游戏
void update(); // 更新游戏状态
void draw(); // 绘制游戏场景
void handleInput(); // 处理玩家输入
void checkCollision(); // 检测碰撞
void gameOver(); // 游戏结束
};
```
4. 在游戏循环中,调用游戏类的方法,处理玩家输入、更新游戏状态、绘制游戏场景等。
```c++
int main() {
Game game;
game.init();
while (true) {
game.handleInput();
game.update();
game.checkCollision();
game.draw();
}
return 0;
}
```
以上就是使用C++面向对象的方式完成飞机大战的基本步骤。当然,具体实现还需要根据实际需求进行调整和完善。
阅读全文
相关推荐
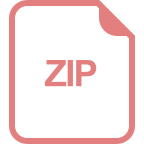
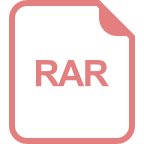
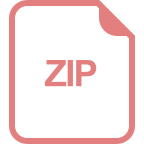









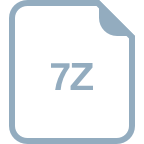
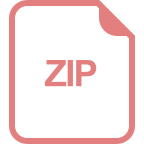