springboot netty tcp客户端
时间: 2023-10-27 07:02:55 浏览: 155
Spring Boot 是一个开源的Java开发框架,用于开发微服务和基于RESTful架构的应用。Netty 是一个用于构建高性能、事件驱动的网络应用程序的Java框架。Netty 提供了TCP 和 UDP 传输协议的支持,因此可以方便地使用Netty构建TCP客户端。
在Spring Boot中使用Netty构建TCP客户端的步骤如下:
1. 在pom.xml文件中添加Netty的依赖项:
```
<dependencies>
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>x.x.x</version>
</dependency>
</dependencies>
```
2. 创建一个TCP客户端的处理器类,继承Netty的SimpleChannelInboundHandler抽象类,重写相应的方法来处理数据。
```
public class TcpClientHandler extends SimpleChannelInboundHandler<String> {
@Override
public void channelActive(ChannelHandlerContext ctx) {
// 在连接建立时发送数据
ctx.writeAndFlush("Hello Server!");
}
@Override
public void channelRead0(ChannelHandlerContext ctx, String message) {
// 处理接收到的数据
System.out.println("Received from server: " + message);
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
// 处理异常情况
cause.printStackTrace();
ctx.close();
}
}
```
3. 创建一个Spring Boot的启动类,并在其中配置Netty的相关参数和创建TCP客户端的Bootstrap实例。
```
@SpringBootApplication
public class TcpClientApplication {
public static void main(String[] args) {
SpringApplication.run(TcpClientApplication.class, args);
}
@Bean
public CommandLineRunner tcpClientRunner() {
return args -> {
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap bootstrap = new Bootstrap()
.group(group)
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) {
ch.pipeline().addLast(new TcpClientHandler());
}
});
ChannelFuture future = bootstrap.connect("localhost", 8888).sync();
future.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
};
}
}
```
以上就是使用Spring Boot和Netty构建TCP客户端的基本步骤。通过以上配置,可以编写相应的业务逻辑来与服务器进行通信,实现相关功能。
阅读全文
相关推荐
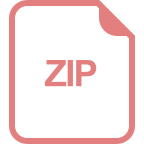
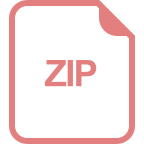




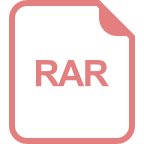
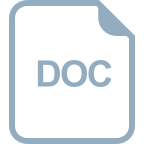









