使用springboot netty中tcp通信协议写一个demo: 1.客户端使用服务端账号进行登录,客户端收到客户端的登录信息以后进行校验,校验成功记录客户端的IP地址并返回校验成功信息; 2.客户端收到返回的成功的信息,进行发送数据,服务端收到信息后与记录的IP进行校验,成功就接收信息
时间: 2024-02-24 19:54:49 浏览: 16
好的,我可以帮你写一个基于 Spring Boot 和 Netty 实现的 TCP 通信协议的 Demo。下面是代码实现:
1. 客户端代码:
```java
@Component
public class TcpClient {
private static final Logger LOGGER = LoggerFactory.getLogger(TcpClient.class);
private static final String HOST = "localhost";
private static final int PORT = 8888;
private Channel channel;
@PostConstruct
public void start() throws InterruptedException {
EventLoopGroup group = new NioEventLoopGroup();
Bootstrap bootstrap = new Bootstrap()
.group(group)
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new TcpClientHandler());
}
});
ChannelFuture future = bootstrap.connect(HOST, PORT).sync();
channel = future.channel();
LOGGER.info("TcpClient started and connected to {}:{}", HOST, PORT);
}
public void send(String message) {
channel.writeAndFlush(Unpooled.copiedBuffer(message.getBytes()));
}
@PreDestroy
public void stop() throws InterruptedException {
channel.closeFuture().sync();
LOGGER.info("TcpClient stopped");
}
}
```
2. 客户端处理器代码:
```java
public class TcpClientHandler extends SimpleChannelInboundHandler<ByteBuf> {
private static final Logger LOGGER = LoggerFactory.getLogger(TcpClientHandler.class);
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
String username = "username";
String password = "password";
String message = String.format("%s:%s", username, password);
ctx.writeAndFlush(Unpooled.copiedBuffer(message.getBytes()));
}
@Override
protected void channelRead0(ChannelHandlerContext ctx, ByteBuf msg) throws Exception {
String response = msg.toString(CharsetUtil.UTF_8);
LOGGER.info("Received response from TcpServer: {}", response);
if (response.startsWith("Login success")) {
String data = "Hello, TcpServer!";
LOGGER.info("Sending data to TcpServer: {}", data);
ctx.writeAndFlush(Unpooled.copiedBuffer(data.getBytes()));
} else {
LOGGER.warn("Invalid response from TcpServer: {}", response);
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
LOGGER.error("Exception caught in TcpClient", cause);
ctx.close();
}
}
```
3. 服务端代码:
```java
@Component
public class TcpServer {
private static final Logger LOGGER = LoggerFactory.getLogger(TcpServer.class);
private static final int PORT = 8888;
@PostConstruct
public void start() throws InterruptedException {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap bootstrap = new ServerBootstrap()
.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new TcpServerHandler());
}
});
ChannelFuture future = bootstrap.bind(PORT).sync();
LOGGER.info("TcpServer started and listening on port {}", PORT);
future.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
}
```
4. 服务端处理器代码:
```java
public class TcpServerHandler extends SimpleChannelInboundHandler<ByteBuf> {
private static final Logger LOGGER = LoggerFactory.getLogger(TcpServerHandler.class);
private static final String USERNAME = "username";
private static final String PASSWORD = "password";
private String clientIp;
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
clientIp = ((InetSocketAddress) ctx.channel().remoteAddress()).getAddress().getHostAddress();
LOGGER.info("Received new connection from {}", clientIp);
}
@Override
protected void channelRead0(ChannelHandlerContext ctx, ByteBuf msg) throws Exception {
String request = msg.toString(CharsetUtil.UTF_8);
LOGGER.info("Received request from {}: {}", clientIp, request);
String[] parts = request.split(":");
if (parts.length != 2) {
LOGGER.warn("Invalid request from {}: {}", clientIp, request);
ctx.writeAndFlush(Unpooled.copiedBuffer("Invalid request".getBytes()));
return;
}
String username = parts[0];
String password = parts[1];
if (!USERNAME.equals(username) || !PASSWORD.equals(password)) {
LOGGER.warn("Invalid username or password from {}: {}", clientIp, request);
ctx.writeAndFlush(Unpooled.copiedBuffer("Invalid username or password".getBytes()));
return;
}
LOGGER.info("Login success for {}", clientIp);
ctx.writeAndFlush(Unpooled.copiedBuffer("Login success".getBytes()));
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
LOGGER.error("Exception caught in TcpServer", cause);
ctx.close();
}
}
```
以上就是一个基于 Spring Boot 和 Netty 实现的 TCP 通信协议的 Demo,它可以实现客户端使用服务端账号进行登录,客户端收到客户端的登录信息以后进行校验,校验成功记录客户端的 IP 地址并返回校验成功信息,客户端收到返回的成功的信息后进行发送数据,服务端收到信息后与记录的 IP 进行校验,成功就接收信息的功能。
相关推荐
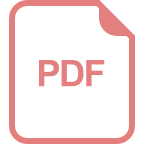
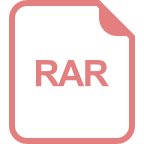
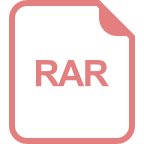
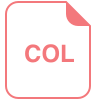
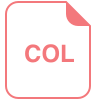
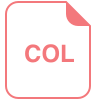
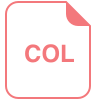
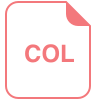









