MATLAB中随机选取矩阵中的坐标
时间: 2024-10-24 07:07:56 浏览: 19
在MATLAB中,你可以使用`randperm`函数配合`sub2ind`函数来随机选择矩阵中的坐标。首先,`randperm`用于生成一个从1到矩阵尺寸元素的排列,然后`sub2ind`将这个排列转换为矩阵中的行、列索引。
下面是一个简单的例子:
```matlab
% 假设你有一个3x3的矩阵A
A = rand(3); % 创建一个3x3的随机矩阵
% 生成一个0到2的随机整数,表示你要取的元素个数
num_elements = 3;
% 使用randperm生成随机的排列
indices = randperm(size(A, 1), num_elements);
% 将排列转换为行、列坐标
row_indices = indices;
col_indices = sub2ind([size(A, 1), size(A, 2)], row_indices, indices);
% 现在row_indices和col_indices就是你随机选中的行和列索引
disp('Randomly selected coordinates:');
disp(row_indices);
disp(col_indices);
```
相关问题
matlab实现:C是城市坐标矩阵,D是距离矩阵,遗传算法解决TSP问题,要求迭代绘制每一次的最优路径
好的,我可以为您提供一个基本的遗传算法实现TSP问题的MATLAB代码。遗传算法是一种优化算法,在TSP问题中可以用来寻找最优路径。以下是代码:
```
% 遗传算法解TSP问题
% C是城市坐标矩阵
% D是距离矩阵
% pop_size是种群数量
% max_gen是最大迭代次数
% pc是交叉概率
% pm是变异概率
function [best_fit, best_route] = ga_tsp(C, D, pop_size, max_gen, pc, pm)
n = size(C, 1); % 城市数量
pop = init_pop(pop_size, n); % 初始化种群
fit = evaluate_pop(pop, D); % 评估种群适应度
best_fit = zeros(max_gen, 1); % 存储每一代的最优适应度
best_route = zeros(n, max_gen); % 存储每一代的最优路径
for t = 1:max_gen
% 选择
parents = select_parents(pop, fit);
% 交叉
offs = crossover(parents, pc);
% 变异
offs = mutation(offs, pm);
% 合并
pop = [pop; offs];
% 评估适应度
fit = evaluate_pop(pop, D);
% 选择下一代
pop = select_pop(pop, fit, pop_size);
% 存储最优适应度和路径
[best_fit(t), idx] = min(fit);
best_route(:, t) = pop(idx, :);
% 绘制路径
plot_tsp(C, best_route(:, t));
end
end
% 初始化种群
function pop = init_pop(pop_size, n)
pop = zeros(pop_size, n);
for i = 1:pop_size
pop(i, :) = randperm(n);
end
end
% 评估种群适应度
function fit = evaluate_pop(pop, D)
pop_size = size(pop, 1);
fit = zeros(pop_size, 1);
for i = 1:pop_size
fit(i) = evaluate_route(pop(i, :), D);
end
end
% 评估路径长度
function len = evaluate_route(route, D)
n = length(route);
len = 0;
for i = 1:n-1
len = len + D(route(i), route(i+1));
end
len = len + D(route(n), route(1));
end
% 选择父代
function parents = select_parents(pop, fit)
pop_size = size(pop, 1);
parents = zeros(pop_size, size(pop, 2));
for i = 1:pop_size
idx1 = randi(pop_size);
idx2 = randi(pop_size);
if fit(idx1) < fit(idx2)
parents(i, :) = pop(idx1, :);
else
parents(i, :) = pop(idx2, :);
end
end
end
% 交叉
function offs = crossover(parents, pc)
pop_size = size(parents, 1);
offs = zeros(pop_size, size(parents, 2));
for i = 1:2:pop_size-1
if rand() < pc
p1 = parents(i, :);
p2 = parents(i+1, :);
[c1, c2] = pmx(p1, p2);
offs(i, :) = c1;
offs(i+1, :) = c2;
else
offs(i, :) = p1;
offs(i+1, :) = p2;
end
end
end
% 部分匹配交叉算子
function [c1, c2] = pmx(p1, p2)
n = length(p1);
c1 = p1;
c2 = p2;
% 随机选取两个交叉点
idx1 = randi(n);
idx2 = randi(n);
while idx1 == idx2
idx2 = randi(n);
end
if idx1 > idx2
tmp = idx1;
idx1 = idx2;
idx2 = tmp;
end
% 部分匹配交叉
for i = idx1:idx2
% 查找相应位置的值
val1 = p1(i);
val2 = p2(i);
j = i + 1;
while j <= n
if c1(j) == val1
c1(j) = val2;
end
if c2(j) == val2
c2(j) = val1;
end
j = j + 1;
end
end
end
% 变异
function offs = mutation(pop, pm)
pop_size = size(pop, 1);
offs = zeros(pop_size, size(pop, 2));
for i = 1:pop_size
if rand() < pm
offs(i, :) = inversion_mutation(pop(i, :));
else
offs(i, :) = pop(i, :);
end
end
end
% 反转变异算子
function route = inversion_mutation(route)
n = length(route);
% 随机选取两个位置
idx1 = randi(n);
idx2 = randi(n);
while idx1 == idx2
idx2 = randi(n);
end
if idx1 > idx2
tmp = idx1;
idx1 = idx2;
idx2 = tmp;
end
% 反转区间
route(idx1:idx2) = fliplr(route(idx1:idx2));
end
% 选择下一代
function pop = select_pop(pop, fit, pop_size)
[~, idx] = sort(fit);
pop = pop(idx(1:pop_size), :);
end
% 绘制路径
function plot_tsp(C, route)
plot(C(route, 1), C(route, 2), 'b.-');
xlim([min(C(:, 1))-1, max(C(:, 1))+1]);
ylim([min(C(:, 2))-1, max(C(:, 2))+1]);
title(sprintf('Total Distance = %f', evaluate_route(route, D)));
drawnow;
end
```
您可以根据需要调整迭代次数、交叉和变异概率等参数。这个代码会在每次迭代后将最优路径绘制出来,您可以观察路径的变化过程。
matlab生成随机模拟车流
在MATLAB中,可以使用随机数函数来生成随机模拟车流。通过rand函数可以生成服从[0,1]上的平均分布的随机数矩阵,其中每个元素都是随机选取的数字。例如,可以使用以下代码生成随机模拟车流:
```
x = rand(1, num_vehicles); % 生成x坐标
y = rand(1, num_vehicles); % 生成y坐标
z = rand(1, num_vehicles); % 生成z坐标
% 判断车辆是否在桥上
on_bridge = (x.^2 + y.^2 <= 1) & (z <= sqrt(1 - x.^2 - y.^2));
% 统计车辆在桥上的数量
num_on_bridge = sum(on_bridge);
```
在上述代码中,我们使用rand函数生成num_vehicles个随机数作为车辆的坐标,然后通过判断车辆的坐标是否在桥上来确定车辆是否在桥上。最后,使用sum函数统计车辆在桥上的数量。
请注意,这只是一个简单的示例,具体的随机模拟车流的实现可能需要根据具体情况进行调整和扩展。
阅读全文
相关推荐
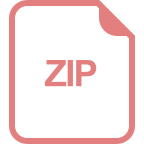
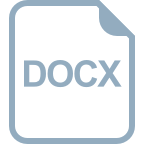
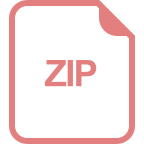
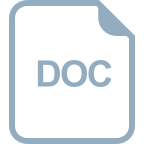
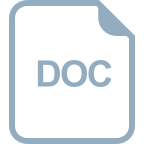
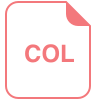
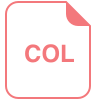
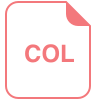
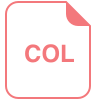
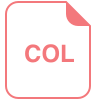







