有两张图片,从各个图片参数,使用python算法比较两张图的相似度
时间: 2024-04-14 19:29:32 浏览: 88
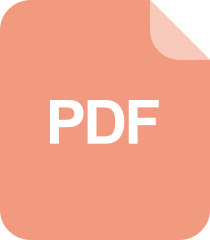
python 比较2张图片的相似度的方法示例
要从两张图片的参数比较它们的相似度,可以使用图像特征提取算法来获取图片的特征向量,然后计算这两个向量之间的相似度。下面是一个使用Python和OpenCV库进行图像特征提取和相似度计算的示例代码:
```python
import cv2
import numpy as np
def calculate_image_similarity(image1, image2):
# 读取两张图片
img1 = cv2.imread(image1)
img2 = cv2.imread(image2)
# 将图片调整为相同的大小
img1 = cv2.resize(img1, (300, 300))
img2 = cv2.resize(img2, (300, 300))
# 使用ORB算法提取特征
orb = cv2.ORB_create()
keypoints1, descriptors1 = orb.detectAndCompute(img1, None)
keypoints2, descriptors2 = orb.detectAndCompute(img2, None)
# 创建一个BFMatcher对象,用于匹配特征
bf = cv2.BFMatcher(cv2.NORM_HAMMING, crossCheck=True)
# 使用BFMatcher进行特征匹配
matches = bf.match(descriptors1, descriptors2)
# 根据匹配结果计算相似度
similarity = len(matches) / max(len(descriptors1), len(descriptors2))
return similarity
# 使用示例
image1 = 'path_to_image1.jpg'
image2 = 'path_to_image2.jpg'
similarity_score = calculate_image_similarity(image1, image2)
print(f"两张图片的相似度为: {similarity_score}")
```
请将 `path_to_image1.jpg` 和 `path_to_image2.jpg` 替换为你实际的图片路径。此示例中使用了ORB(Oriented FAST and Rotated BRIEF)算法进行特征提取,然后使用BFMatcher进行特征匹配,并根据匹配结果计算相似度。你也可以尝试其他的特征提取算法,如SIFT(Scale-Invariant Feature Transform)或SURF(Speeded-Up Robust Features)。
阅读全文
相关推荐
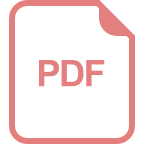
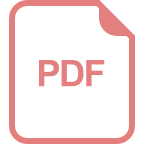















