从键盘读入若于个整数,建一个整数单链表,并完成下列操作: (1) 打印该链表; (2) 在链表中插入一个结点,结点的数据域从键盘读入, 打印该链表; (3) 在链表中删除一个结点,被删结点的位置从键盘读入,打印该链表
时间: 2024-09-20 21:03:30 浏览: 41
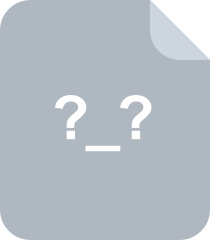
创建一个数据类型为T的链表类模板List,实现以下成员函数的模拟测试.cpp

在编程中,创建一个整数单链表并完成上述操作通常涉及以下几个步骤:
1. **初始化链表**:首先,你需要创建一个`Node`类,它包含整数值和指向下一个节点的指针。然后创建一个`LinkedList`类,其中维护头节点。
```python
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
```
2. **输入和添加元素**:
- 定义一个方法`insert_at_end`用于向链表末尾添加新节点,以及一个`read_input`方法从键盘读取整数。
```python
def read_input(self):
num = int(input("请输入一个整数:"))
return num
def insert_at_end(self, node):
if not self.head:
self.head = Node(node)
else:
current = self.head
while current.next:
current = current.next
current.next = Node(node)
```
3. **打印链表**:
- 创建一个`print_list`方法遍历整个链表并打印数据。
```python
def print_list(self):
current = self.head
while current:
print(current.data, end=" -> ")
current = current.next
print("None")
```
4. **删除节点**:
- 定义一个`delete_node`方法,接受位置作为参数,找到并移除对应位置的节点。注意,需要处理边界条件如第一个节点和列表为空的情况。
```python
def delete_node(self, position):
if self.head is None or position <= 0:
return
if position == 1:
self.head = self.head.next
else:
current = self.head
for _ in range(position - 1):
current = current.next
if current and current.next:
current.next = current.next.next
```
5. **完整实现**:
- 将上述方法组合起来,按照所需顺序依次执行。
```python
def main():
my_list = LinkedList()
# 插入节点
input_count = int(input("请输入你要插入的整数数量:"))
for _ in range(input_count):
my_list.insert_at_end(my_list.read_input())
# 打印链表
my_list.print_list()
# 删除节点
pos_to_delete = int(input("请输入要删除的节点位置:"))
my_list.delete_node(pos_to_delete)
# 再次打印链表
my_list.print_list()
if __name__ == "__main__":
main()
```
阅读全文
相关推荐
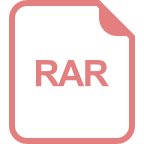
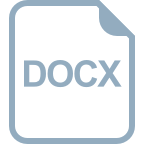
















