用C++建立单链表,实现插入元素、删除元素、查询元素、求表长度
时间: 2024-06-17 12:02:35 浏览: 132
在C++中,可以使用结构体和指针来建立单链表,并实现插入元素、删除元素、查询元素和求表长度的功能。
首先,定义一个结构体表示链表的节点,包含一个数据成员和一个指向下一个节点的指针:
```cpp
struct Node {
int data;
Node* next;
};
```
接下来,可以定义一个链表类,其中包含头节点指针和一些操作函数:
```cpp
class LinkedList {
private:
Node* head; // 头节点指针
public:
LinkedList() {
head = nullptr; // 初始化为空链表
}
// 插入元素
void insert(int value) {
Node* newNode = new Node; // 创建新节点
newNode->data = value;
newNode->next = nullptr;
if (head == nullptr) {
head = newNode; // 如果链表为空,直接将新节点设为头节点
} else {
Node* current = head;
while (current->next != nullptr) {
current = current->next; // 找到链表的最后一个节点
}
current->next = newNode; // 将新节点插入到最后
}
}
// 删除元素
void remove(int value) {
if (head == nullptr) {
return; // 链表为空,直接返回
}
if (head->data == value) {
Node* temp = head;
head = head->next;
delete temp; // 删除头节点
} else {
Node* current = head;
while (current->next != nullptr && current->next->data != value) {
current = current->next; // 找到要删除节点的前一个节点
}
if (current->next != nullptr) {
Node* temp = current->next;
current->next = current->next->next;
delete temp; // 删除节点
}
}
}
// 查询元素
bool search(int value) {
Node* current = head;
while (current != nullptr) {
if (current->data == value) {
return true; // 找到了该元素
}
current = current->next;
}
return false; // 没有找到该元素
}
// 求表长度
int length() {
int count = 0;
Node* current = head;
while (current != nullptr) {
count++;
current = current->next;
}
return count;
}
};
```
使用上述链表类,可以进行插入、删除、查询和求表长度的操作。以下是相关问题:
阅读全文
相关推荐

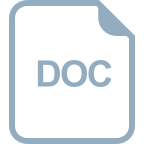
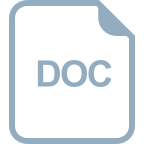
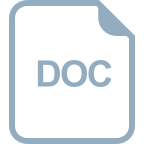
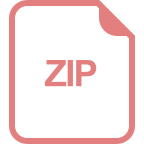
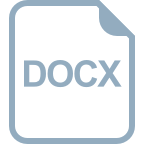
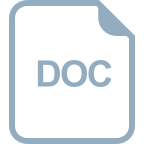
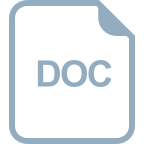
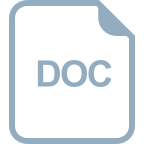
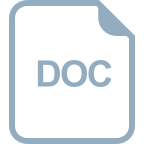




