请用booth算法实现整数的乘法,用C语言实现
时间: 2024-12-17 20:42:08 浏览: 11
Booth算法是一种高效的二进制乘法算法,特别适合在硬件上实现,因为它减少了乘法所需的逻辑门。下面是使用C语言实现Booth算法的基本步骤,这里仅给出核心部分的代码示例,实际应用中可能会包含更多的错误检查和边界条件处理。
```c
#include <stdio.h>
typedef struct {
int bits[4]; // 只使用最少两位存储一个数,实际应用需根据位宽调整
int carry; // 进位标志
} BoothMultiplicand;
void boothMultiply(int multiplicand1, int multiplicand2) {
BoothMultiplicand mult1 = { { multiplicand1 & 0x3, multiplicand1 >> 2 }, 0 };
BoothMultiplicand mult2 = { { multiplicand2 & 0x3, multiplicand2 >> 2 }, 0 };
while (mult1.bits[0] != 0 || mult2.bits[0] != 0) {
BoothMultiplicand tempMult1 = mult1;
BoothMultiplicand tempMult2 = mult2;
// 当前位的相乘
BoothMultiplicand product = { {tempMult1.bits[0] & tempMult2.bits[0], 0}, 0 };
// 下一位的进位影响当前位的计算
if (tempMult1.carry != 0) {
product.bits[0] += tempMult2.bits[1];
tempMult1.carry = 1;
}
if (tempMult2.carry != 0) {
product.bits[0] += tempMult1.bits[1];
tempMult2.carry = 1;
}
// 更新乘积
mult1 = product;
mult2 = { { tempMult2.bits[0], tempMult2.carry }, { tempMult1.bits[1], temp1.carry } }; // 交换左右位以准备下一轮迭代
}
// 结果是倒序的,所以反转数组
for (int i = 0, j = mult1.bits[0]; i < sizeof(mult1.bits)/sizeof(mult1.bits[0]) - 1; i++, j = mult1.bits[i])
mult1.bits[i] = j;
// 把结果转换回普通的二进制整数
int result = 0;
for (int i = 0; i < sizeof(mult1.bits)/sizeof(mult1.bits[0]); ++i) {
result <<= 2;
result |= mult1.bits[i];
}
printf("Booth乘法的结果: %d\n", result);
}
int main() {
int multiplicand1 = 14; // 0b1110
int multiplicand2 = 6; // 0b0110
boothMultiply(multiplicand1, multiplicand2);
return 0;
}
```
注意这只是一个简化版本,实际项目中需要考虑更多边缘情况和性能优化。
阅读全文
相关推荐
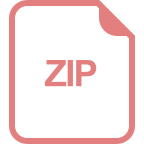
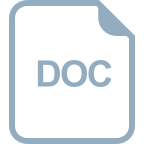
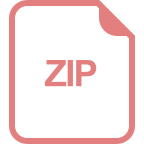



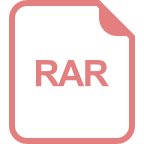
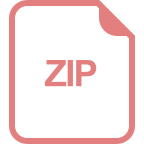
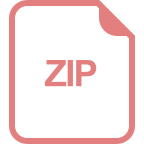
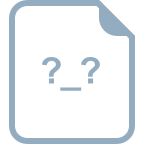
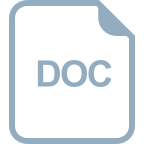
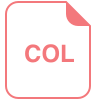
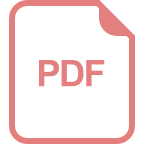