c语言实现booth算法,输入格式为: 0.110111 -0.101110(中间用空格间隔:0.110111(空格) -0.101110) 输出为; -0.100111100010(小数点后的位数为两个输入小数点后位数之和)
时间: 2024-12-17 16:56:25 浏览: 14
Booth's Algorithm是一种用于二进制补码小数乘法的快速算法。在C语言中实现这个算法,你需要编写一系列函数来处理符号、截断和位移操作。以下是简化的步骤:
1. 首先,判断输入的小数是否为正数。如果是负数,需要将其转换为补码形式,并记录下符号。
```c
int sign = 1; // 初始化为正数
if (input[0] == '-') {
sign = -1;
input++; // 跳过第一个负号字符
}
```
2. 将输入的二进制小数转换为二进制整数表示,便于处理。
```c
int fraction = 0;
for (int i = 0; i < strlen(input); i++) {
fraction <<= 1; // 左移一位
if (input[i] == '1') {
fraction |= 1; // 如果当前位是1,则置位
}
}
```
3. 使用Booth编码,将整数部分转换为两倍长度的二进制序列。
```c
int BoothCode[64]; // 假设最多有32位
int index = 0;
while (fraction > 0) {
if ((fraction & 1) != 0) { // 如果原数最低位是1
BoothCode[index++] = 1; // 添加到编码
}
fraction >>= 1; // 右移一位
BoothCode[index++] = 0; // 添加0
}
```
4. 对Booth编码进行逐次相乘,然后进行位移。
```c
for (int i = 0; i < BoothCode.length / 2; i++) {
// 计算 Booth 算法的结果
int product = BoothCode[i] * fraction + BoothCode[i+1];
product <<= 1; // 双倍位移
BoothCode[i*2] = product & 0x00000001; // 第一个结果位
BoothCode[i*2+1] = product >> 1; // 第二个结果位
}
```
5. 最后,根据符号恢复原始结果并返回。
```c
// 结果转换回小数表示
char result[2*(strlen(input) + 1)];
result[0] = sign == 1 ? '+' : '-'; // 根据符号添加'
for (int i = 0; i < BoothCode.length; i += 2) {
result[strlen(input)] = BoothCode[i] ^ BoothCode[i+1]; // 抵消偶数位置的0
result[strlen(input)+1] = BoothCode[i+1]; // 保留奇数位置
result[strlen(input)] = BoothCode[i]; // 增加额外的零,保证总位数正确
strlen(input)++;
}
printf("%s%.0f", result, strlen(result)-1);
```
阅读全文
相关推荐
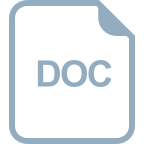
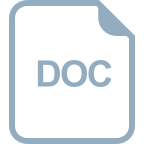
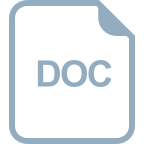
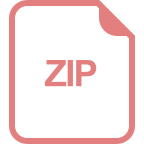

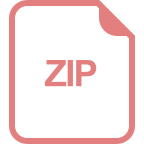
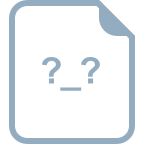
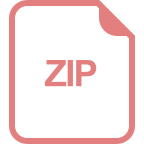
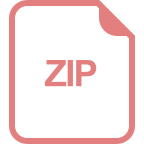
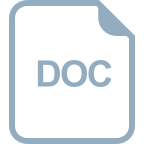



