stm32 PC6外部中断代码
时间: 2024-08-14 19:00:52 浏览: 58
STM32 微控制器支持多种类型的中断,包括外部中断(EXTI)。外部中断允许处理器响应来自外部设备(如按钮、传感器等)的事件,并通过中断处理程序执行相应的操作。
### STM32 中断配置基本步骤:
#### 步骤 1: 初始化 GPIO 引脚作为外部中断触发端口
首先,在 `main.c` 或者 `stm32f4xx_hal_misr.c` 文件中初始化GPIO引脚并设置其模式。例如,如果你打算使用PA0作为外部中断触发信号:
```c
#include "stm32f4xx_hal.h"
void init_GPIO()
{
GPIO_InitTypeDef GPIO_InitStruct = {0};
/* Configure GPIO Ports */
__HAL_RCC_GPIOA_CLK_ENABLE(); // Enable GPIO Clock
GPIO_InitStruct.Pin = GPIO_PIN_0; // Pin 0 of GPIOA
GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; // Set as rising edge interrupt
GPIO_InitStruct.Pull = GPIO_NOPULL;
HAL_GPIO_Init(GPIOA, &GPIO_InitStruct);
}
```
#### 步骤 2: 配置 EXTI 线路
接下来,需要配置EXTI线路上的中断服务功能和对应的中断向量:
```c
void init_EXTI()
{
NVIC_InitTypeDef NVIC_InitStructure;
/* Enable the EXTI peripheral */
__HAL_RCC_EXTI_CLK_ENABLE();
/* EXTI line configuration */
EXTI_InitTypeDef EXTIInitStructure = {0};
EXTIInitStructure.Line = EXTI_LINE_PA0; // Example for PA0
EXTIInitStructure mode = EXTI_Mode_Interrupt;
EXTIInitStructure.Trigger = EXTI_Trigger_Rising;
HAL_NVIC_SetPriority(EXTI0_IRQn, 5, 0);
HAL_NVIC_EnableIRQ(EXTI0_IRQn);
HAL_EXTI_Init(&EXTIInitStructure);
}
```
#### 步骤 3: 实现中断服务函数 (ISR)
创建一个中断服务函数来处理外部中断:
```c
static void EXTI0_IRQHandler(void)
{
static uint8_t flag EXTI0_flag = 0;
if (__HAL_GPIO_GET_IT_SOURCE(GPIOA, GPIO_PIN_0)) {
// Handle external event here
if (!flag) {
flag = 1;
// Do something when an event occurs on PA0.
// For example, print a message or change system state.
printf("External event detected on PA0.\n");
}
else {
flag = 0;
// Reset the flag after handling the event to prevent duplicate calls in rapid succession
}
} else {
flag = 0;
}
__HAL_GPIO_CLEAR_IT(GPIOA, GPIO_PIN_0); // Clear the interrupt source
}
// In main() function call this function once after initializing EXTI and GPIO
HAL_NVIC_StartTick();
```
### 关联问题:
1. **如何在STM32中配置GPIO作为外部中断触发端口?**
2. **STM32的EXTI中断是如何工作的?**
3. **在STM32项目中加入外部中断的完整步骤是什么?**
阅读全文
相关推荐
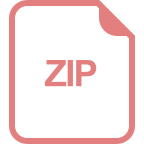
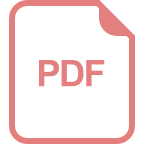
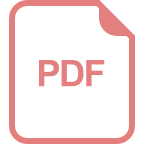



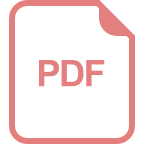
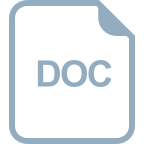










