c++ vector取交集、并集
时间: 2023-08-19 13:13:10 浏览: 245
回答: 要计算两个vector的交集和并集,可以使用STL中的算法和容器操作。首先,我们需要包含头文件<set>和<algorithm>。然后,我们可以使用set容器来存储vector中的元素,因为set会自动去重。接下来,我们可以使用set_intersection算法来计算两个vector的交集,使用set_union算法来计算两个vector的并集。
下面是一个示例代码,展示了如何计算两个vector的交集和并集:
```cpp
#include <iostream>
#include <vector>
#include <set>
#include <algorithm>
int main() {
int a[6] = {1, 2, 3, 4, 5, 6};
int b[4] = {4, 5, 6, 7};
std::vector<int> vectorA(a, a + 6);
std::vector<int> vectorB(b, b + 4);
std::set<int> setA(vectorA.begin(), vectorA.end());
std::set<int> setB(vectorB.begin(), vectorB.end());
std::set<int> intersection;
std::set_intersection(setA.begin(), setA.end(), setB.begin(), setB.end(), std::inserter(intersection, intersection.begin()));
std::set<int> unionSet;
std::set_union(setA.begin(), setA.end(), setB.begin(), setB.end(), std::inserter(unionSet, unionSet.begin()));
std::cout << "Intersection: ";
for (auto it = intersection.begin(); it != intersection.end(); ++it) {
std::cout << *it << " ";
}
std::cout << std::endl;
std::cout << "Union: ";
for (auto it = unionSet.begin(); it != unionSet.end(); ++it) {
std::cout << *it << " ";
}
std::cout << std::endl;
return 0;
}
```
这段代码首先将两个数组转换为vector,然后使用set容器存储vector中的元素。接下来,使用set_intersection算法计算两个set的交集,并使用set_union算法计算两个set的并集。最后,通过遍历set来输出交集和并集的元素。
希望这个例子能够帮助你理解如何计算vector的交集和并集。
阅读全文
相关推荐
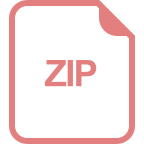

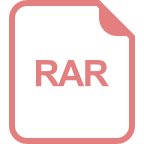
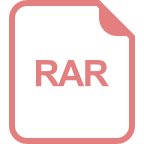
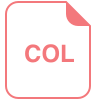











