java将html转word并将图片打进word内,离线可看
时间: 2023-09-07 12:01:50 浏览: 275
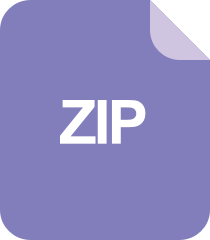
java将word转图片转pdf_jacob.zip
将HTML转为Word并将图片插入Word文档的方法,可以使用Java的Apache POI库来实现。
首先,你需要下载并导入Apache POI库的相关JAR文件。可以从Apache官方网站上找到最新的版本。
接下来,你可以使用以下步骤来实现HTML转Word并插入图片:
1. 创建一个新的Word文档对象并打开它。
```java
XWPFDocument document = new XWPFDocument();
```
2. 读取HTML内容并将其转换为Word格式。
```java
String html = "<html><body><h1>Hello, World!</h1></body></html>";
InputStream inputStream = new ByteArrayInputStream(html.getBytes());
HWPFDocumentCore wordDoc = WordToHtmlUtils.loadHtml(inputStream);
WordToHtmlConverter wordToHtmlConverter = new WordToHtmlConverter(
DocumentBuilderFactory.newInstance().newDocumentBuilder().newDocument()
);
wordToHtmlConverter.processDocument(wordDoc);
org.w3c.dom.Document htmlDocument = wordToHtmlConverter.getDocument();
ByteArrayOutputStream out = new ByteArrayOutputStream();
DOMSource domSource = new DOMSource(htmlDocument);
StreamResult streamResult = new StreamResult(out);
TransformerFactory tf = TransformerFactory.newInstance();
Transformer serializer = tf.newTransformer();
serializer.setOutputProperty(OutputKeys.ENCODING, "UTF-8");
serializer.setOutputProperty(OutputKeys.INDENT, "yes");
serializer.setOutputProperty(OutputKeys.METHOD, "html");
serializer.transform(domSource, streamResult);
String content = new String(out.toByteArray());
```
3. 使用正则表达式提取HTML中的图片链接。
```java
Pattern pattern = Pattern.compile("<img[^>]+src=\"([^\"]+)\"[^>]*>");
Matcher matcher = pattern.matcher(content);
```
4. 遍历图片链接,并将图片插入Word文档中。
```java
while (matcher.find()) {
String imageUrl = matcher.group(1);
InputStream imageStream = new FileInputStream(imageUrl);
String[] imgExt = imageUrl.split("\\.");
String imgType = imgExt[imgExt.length - 1];
int imgFormat = XWPFDocument.PICTURE_TYPE_PNG; // 默认为PNG格式图片
if (imgType.equalsIgnoreCase("jpg") || imgType.equalsIgnoreCase("jpeg")) {
imgFormat = XWPFDocument.PICTURE_TYPE_JPEG;
}
XWPFParagraph paragraph = document.createParagraph();
XWPFRun run = paragraph.createRun();
int width = Units.toEMU(300); // 设置图片宽度
int height = Units.toEMU(200); // 设置图片高度
run.addPicture(imageStream, imgFormat, "image", width, height);
imageStream.close();
}
```
5. 保存Word文档。
```java
FileOutputStream outputStream = new FileOutputStream("output.docx");
document.write(outputStream);
outputStream.close();
```
通过以上步骤,你可以将HTML转换为Word并插入图片,最后将结果保存为一个可离线查看的Word文档。在代码中,你需要将图片链接替换为实际的图片文件路径,并根据需要调整图片的尺寸和格式。
阅读全文
相关推荐
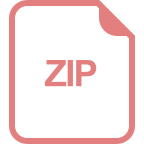
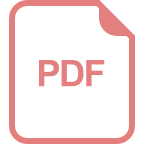















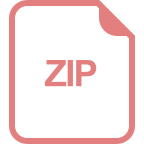