写出一个完整的C语言代码输入一个用户提供的字符串。 调用标识符识别函数和整数识别函数。 输出识别到的结果:如果为标识符,显示"标识符:[识别的值]";若为整数,显示"整数:[识别的值]";否则,输出"错误:无法识别的单词"。
时间: 2024-10-09 14:10:06 浏览: 42
```c
#include <stdio.h>
#include <ctype.h> // 包含用于检查字符类型的头文件
// 定义状态枚举,表示识别过程中的状态
typedef enum {
STATE_START,
STATE_IDENTIFIER,
STATE_INTEGER
} RecognitionState;
// 标识符识别函数
char *detect_identifier(const char *str, int *start) {
while (*str && isalpha(*str)) { // 只识别字母
str++;
}
*start = (int)(str - str);
return str;
}
// 整数识别函数
double detect_integer(const char *str, int *start) {
while (*str && isdigit(*str)) { // 遍历数字字符
str++;
}
*start = (int)(str - str);
return atof(str); // 将字符串转换为double类型
}
// 主函数
int main() {
char input[100];
printf("请输入一个字符串: ");
fgets(input, sizeof(input), stdin); // 获取用户输入
input[strcspn(input, "\n")] = '\0'; // 清除换行符
size_t len = strlen(input);
char *pos = input;
int identifier_start = 0;
double integer_start = 0;
if (isdigit(input[0])) { // 如果首字符是数字,直接认为是整数
integer_start = 1;
} else {
pos = detect_identifier(pos, &identifier_start);
if (!isalnum(*pos) || isspace(*pos)) { // 若不是标识符或紧接着不是字母/数字,视为错误
printf("错误:无法识别的单词\n");
return 1;
}
pos = detect_integer(pos + identifier_start, &integer_start);
}
if (integer_start > 0) {
printf("整数:%.2f\n", integer_start);
} else if (identifier_start > 0) {
printf("标识符:%.*s\n", identifier_start, input);
} else {
printf("错误:无法识别的单词\n");
}
return 0;
}
```
这个C语言程序首先获取用户输入的字符串,然后尝试通过`detect_identifier`函数找到第一个标识符的起始位置,接着通过`detect_integer`函数寻找整数的起始位置。最后根据找到的位置,判断是标识符还是整数,并输出相应的结果。如果字符串不符合任何条件,则输出错误消息。
阅读全文
相关推荐
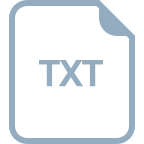
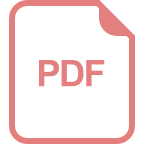
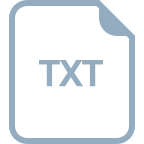
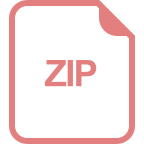
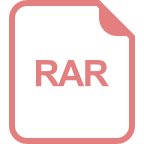
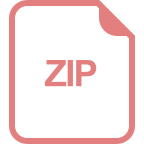
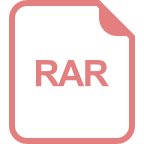
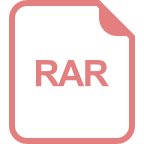
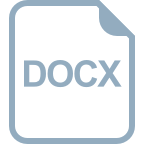
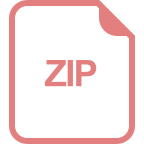
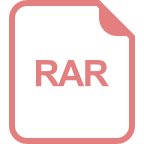
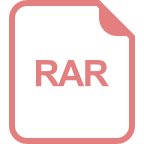
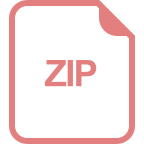
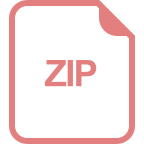
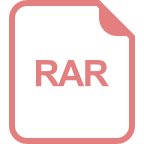
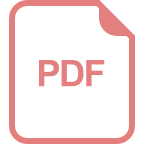