数据结构线性表获取元素位置
时间: 2024-10-21 15:10:34 浏览: 25
数据结构中的线性表,如数组、链表等,获取元素的位置通常取决于它们的具体实现。以下是两种常见情况:
1. **数组**:对于数组,元素的位置由其索引表示,索引是从0开始的整数。例如,在C++或Python中,你可以通过元素的下标直接访问数组中的元素。获取元素位置(即索引)通常非常快,时间复杂度为O(1)。
```cpp
int index = findElement(array, target); // 假设findElement是一个查找函数
cout << "Element at position " << index << " is found." << endl;
```
2. **链表**:链表中的元素没有固定的位置,每个节点包含指向下一个节点的指针。如果要获取某个元素的位置,你需要遍历整个链表,直到找到目标元素或遍历到链表末尾。这通常的时间复杂度为O(n),因为最坏的情况是需要检查所有节点。
```cpp
struct ListNode {
int val;
ListNode *next;
};
ListNode* head = ...; // 假设head是链表头
ListNode* current = head;
while (current != nullptr && current->val != target) {
current = current->next;
}
if (current == nullptr) {
cout << "Element not found in the list." << endl;
} else {
cout << "Element found at position: " << distance(head, current) << endl;
}
```
在上述例子中,`distance(head, current)`计算了从头节点到目标节点的实际位置。
阅读全文
相关推荐



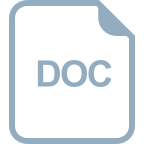

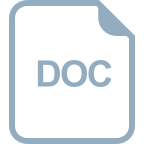
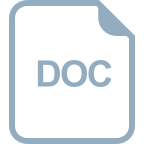
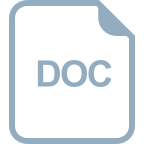
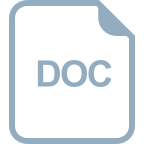


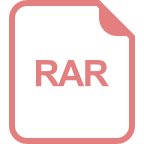
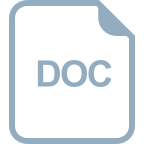





