c++实现一个能进行稀疏矩阵基本运算的运算器
时间: 2024-12-14 07:28:21 浏览: 7
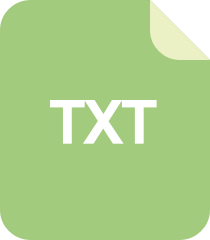
稀疏矩阵运算器加法和减法运算(三元组)

在C++中,要实现一个支持稀疏矩阵的基本运算(如加法、减法和乘法)的运算器,我们可以使用邻接表或者压缩稀疏行(CSR)这样的数据结构来存储矩阵元素,因为它们可以有效地处理稀疏矩阵,节省内存空间。
首先,我们需要定义两个类,一个是`SparseMatrix`,包含矩阵的数据和表示其稀疏性的数据结构;另一个是运算符类`SparseMatrixOperator`,实现基本的运算操作。
以下是一个简化的实现示例:
```cpp
#include <vector>
#include <unordered_map>
// 使用邻接列表存储稀疏矩阵
class SparseMatrix {
public:
std::vector<int> row;
std::vector<int> col;
std::vector<double> val;
// 添加元素
void addElement(int row_i, int col_i, double val_i) {
row.push_back(row_i);
col.push_back(col_i);
val.push_back(val_i);
}
// 其他方法如获取元素、大小等...
};
// 运算符类
class SparseMatrixOperator {
public:
static SparseMatrix operator+(const SparseMatrix& mat1, const SparseMatrix& mat2);
static SparseMatrix operator-(const SparseMatrix& mat1, const SparseMatrix& mat2);
static SparseMatrix operator*(const SparseMatrix& mat1, const SparseMatrix& mat2); // 仅对方阵有效
private:
// 实现加法、减法和乘法的具体算法
// 使用并查集合并行(加法),更新对应位置的值(减法),矩阵相乘则需要额外复杂度(乘法)
// 这里省略了具体的算法实现细节
};
// 示例运算符实现
SparseMatrix SparseMatrixOperator::operator+(const SparseMatrix& mat1, const SparseMatrix& mat2) {
... // 实现矩阵加法逻辑
}
// 类似地实现减法和乘法运算
```
阅读全文
相关推荐
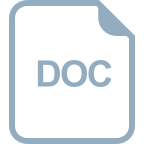
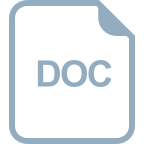
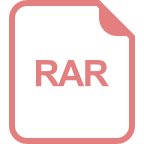
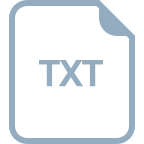
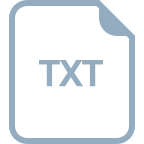
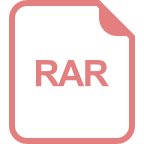
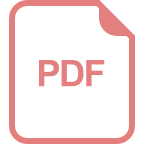
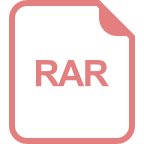
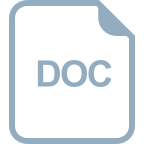
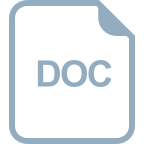
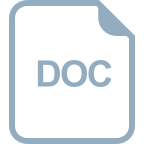

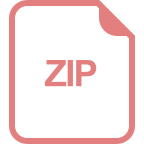
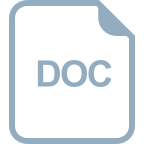

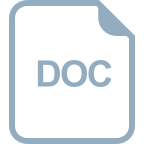
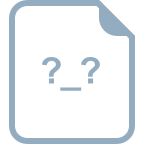
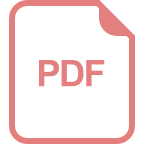