用低版本的c++定义一个复数类,用运算符重载的方法实现复数的输入,输出,基本的数学运算
时间: 2024-10-18 16:21:27 浏览: 41
在C++中,我们可以定义一个简单的复数类(Complex),利用运算符重载来支持复数的输入、输出以及基本的数学运算。以下是一个基础的示例:
```cpp
#include <iostream>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
// 构造函数接受实部和虚部
Complex(double r = 0.0, double i = 0.0) : real(r), imag(i) {}
// 运算符重载 - 输入
friend istream& operator>>(istream& in, Complex& c) {
in >> c.real >> c.imag;
return in;
}
// 运算符重载 - 输出
friend ostream& operator<<(ostream& out, const Complex& c) {
out << c.real << "+" << c.imag << "i";
return out;
}
// 加法运算符重载
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
// 减法运算符重载
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
// 数学乘法运算符重载
Complex operator*(const Complex& other) const {
return Complex(real * other.real - imag * other.imag,
real * other.imag + imag * other.real);
}
};
int main() {
Complex c1(2.5, 3.7);
Complex c2(4.2, -1.8);
cout << "c1 = " << c1 << endl;
cin >> c1; // 输入复数
cout << "Input: " << c1 << endl;
Complex result = c1 + c2;
cout << "c1 + c2 = " << result << endl;
Complex product = c1 * c2;
cout << "c1 * c2 = " << product << endl;
return 0;
}
```
在这个例子中,我们定义了输入(`operator>>`)、输出(`operator<<`)以及加法(`+`)、减法(`-`)和乘法(`*`)运算符,使得复杂类的行为类似于标准的数字类型。在`main`函数中展示了如何创建复数对象并进行操作。
阅读全文
相关推荐




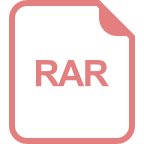













