用链表完整实现栈的基本操作。 Node STACK {int data; node *link;}; Node *top=NULL;
时间: 2024-10-17 10:14:25 浏览: 44
要使用链表实现栈(Stack)的基本操作,你需要定义一个`Node`结构体,包含数据成员`data`和指向下一个节点的指针`link`。这里,`top`是一个指向栈顶元素的指针,初始化为`NULL`,表示栈为空。
以下是链表栈的主要操作:
1. **栈的初始化**:
```cpp
struct Node {
int data;
Node* link;
};
// 初始化空栈
Node* top = NULL;
```
2. **入栈(Push)**:
- 将新元素添加到链表头部(因为链表的顶部是新插入的地方),并更新`top`指针。
```cpp
void push(int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->link = top;
top = newNode;
}
```
3. **出栈(Pop)**:
- 如果栈不为空,删除栈顶元素,返回其值,然后调整`top`指针。
```cpp
int pop() {
if (top == NULL) {
throw std::runtime_error("Stack is empty");
}
int data = top->data;
Node* temp = top;
top = top->link;
delete temp;
return data;
}
```
4. **查看栈顶元素(Top)**:
- 只读操作,返回但不移除栈顶元素的值。如果栈为空,则抛出异常。
```cpp
int topElement() const {
if (top == NULL) {
throw std::runtime_error("Stack is empty");
}
return top->data;
}
```
5. **判断栈是否为空(IsEmpty)**:
```cpp
bool isEmpty() const {
return top == NULL;
}
```
6. **销毁栈(Clear 或者 Delete Stack)**:
- 清理所有节点,释放内存。这通常涉及到遍历整个链表并调用每个节点的析构函数。
```cpp
void clear() {
Node* current = top;
while (current != NULL) {
Node* next = current->link;
delete current;
current = next;
}
top = NULL;
}
```
阅读全文
相关推荐
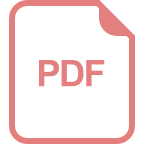
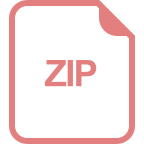
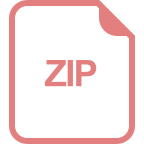















