栈 Stack 是一种先入后出的数据结构,最先入栈的元素称为栈底,最后入栈的元素称为栈顶。为了方便,可用 node.hpp 中的链表结构实现栈,并用链表头指向栈顶元素。 根据下述定义,请实现一个类模板 Stack ,使其可以保存不同类型的数据。#include "genericStack.hpp" #include <iostream> using namespace std; int main() //程序EX6_4.cpp { Stack<int> stack; // 实例化一个保存int型元素的栈 for (int i = 1; i < 9; i++) // 向栈中压入8个元素 stack.push(i); while (!stack.isEmpty()) { // 栈不为空时循环 cout << stack.getTop() << " "; // 显示栈顶元素 stack.pop(); // 弹出栈顶元素 } return 0; }
时间: 2024-02-14 08:12:24 浏览: 69
抱歉,你的代码中缺少了 Stack 类的定义。以下是一个可能的实现:
genericStack.hpp
```c++
#ifndef GENERICSTACK_HPP_
#define GENERICSTACK_HPP_
template <typename ElementType>
class Stack {
public:
Stack();
~Stack();
void push(ElementType obj);
ElementType pop();
bool isEmpty();
int size();
ElementType getTop();
private:
struct Node {
ElementType data;
Node *next;
Node(ElementType d, Node *n = NULL) {
data = d;
next = n;
}
};
Node *top;
int count;
};
template <typename ElementType>
Stack<ElementType>::Stack() {
top = NULL;
count = 0;
}
template <typename ElementType>
Stack<ElementType>::~Stack() {
while (!isEmpty()) {
pop();
}
}
template <typename ElementType>
void Stack<ElementType>::push(ElementType obj) {
Node *newNode = new Node(obj, top);
top = newNode;
count++;
}
template <typename ElementType>
ElementType Stack<ElementType>::pop() {
if (isEmpty()) {
throw "Stack is empty!";
}
ElementType result = top->data;
Node *oldTop = top;
top = top->next;
delete oldTop;
count--;
return result;
}
template <typename ElementType>
bool Stack<ElementType>::isEmpty() {
return count == 0;
}
template <typename ElementType>
int Stack<ElementType>::size() {
return count;
}
template <typename ElementType>
ElementType Stack<ElementType>::getTop() {
if (isEmpty()) {
throw "Stack is empty!";
}
return top->data;
}
#endif /* GENERICSTACK_HPP_ */
```
main.cpp
```c++
#include "genericStack.hpp"
#include <iostream>
using namespace std;
int main()
{
Stack<int> stack;
for (int i = 1; i < 9; i++) {
stack.push(i);
}
while (!stack.isEmpty()) {
cout << stack.getTop() << " ";
stack.pop();
}
return 0;
}
```
阅读全文
相关推荐

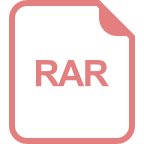



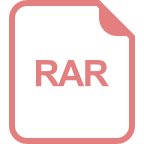




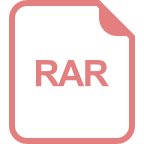






