1. 已知链表结构为:typedef struct Node{int data; struct Node *next;}Node;编写函数实现:合并两个有序链表,使之仍然有序。函数原型为:Node * merge(Node*h1,Node*h2); 2. 2. 已知链表结构为:typedef struct Node{int data; struct Node *next;}Node;编写函数实现:在链表的倒数第k个位置插入元素x(即x成为链表的倒数第k个元素),插入成功返回链表头指针(链表第一个结点的地址),不成功返回NULL(假设链表结点都在堆区)。函数原型为:Node * fun(Node *h, int x, int k); 3. 设链表结点结构已经给出,试编写链表插入函数Insert将实现链表末尾添加数据,再编写函数Print,实现链表中链表数据打印,编写Destroy函数,实现链表的销毁释放,并在main函数中写出完整的调用过程。 typedef struct node { char Name[20]; struct node *Next; }Node;
时间: 2024-03-12 08:06:02 浏览: 17
1. 合并两个有序链表的函数实现如下:
```
Node* merge(Node* h1, Node* h2) {
if (!h1) {
return h2;
}
if (!h2) {
return h1;
}
if (h1->data < h2->data) {
h1->next = merge(h1->next, h2);
return h1;
} else {
h2->next = merge(h1, h2->next);
return h2;
}
}
```
2. 在链表的倒数第k个位置插入元素x的函数实现如下:
```
Node* fun(Node* h, int x, int k) {
Node* p = h;
Node* q = h;
for (int i = 0; i < k; i++) {
if (!q) {
return NULL;
}
q = q->next;
}
while (q) {
p = p->next;
q = q->next;
}
Node* node = (Node*) malloc(sizeof(Node));
node->data = x;
node->next = p->next;
p->next = node;
return h;
}
```
3. 插入、打印和销毁链表的函数实现如下:
```
void Insert(Node** head, char name[]) {
Node* node = (Node*) malloc(sizeof(Node));
strcpy(node->Name, name);
node->Next = NULL;
if (*head == NULL) {
*head = node;
} else {
Node* p = *head;
while (p->Next) {
p = p->Next;
}
p->Next = node;
}
}
void Print(Node* head) {
Node* p = head;
while (p) {
printf("%s ", p->Name);
p = p->Next;
}
printf("\n");
}
void Destroy(Node** head) {
Node* p = *head;
while (p) {
Node* q = p->Next;
free(p);
p = q;
}
*head = NULL;
}
```
完整调用过程如下:
```
int main() {
Node* head = NULL;
Insert(&head, "Alice");
Insert(&head, "Bob");
Insert(&head, "Charlie");
Print(head);
Destroy(&head);
return 0;
}
```
相关推荐
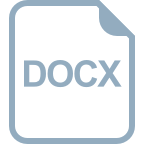
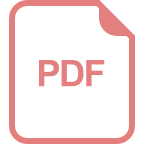
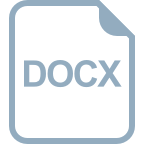














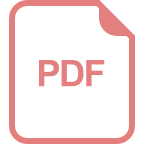