实现单向链表 链表节点类型如下:typedef char datatype;struct node f datatype data; struct node *next;};编写程序实现单向链表的建立八读取和删除函数,在主函数中选择执行。
时间: 2024-09-17 20:01:30 浏览: 54
实现单向链表通常涉及以下几个步骤:
1. 定义链表节点结构体:首先,创建一个结构体`node`,包含数据域`data`(在这里是字符类型`datatype`),以及指向下一个节点的指针`next`。
```c
typedef char datatype;
struct node {
datatype data;
struct node* next;
};
```
2. 创建链表:链表的头结点需要初始化为空,表示初始状态无元素。
```c
struct node* createList() {
struct node* head = NULL;
return head;
}
```
3. 插入元素到链表:函数`insert`接受一个新数据值和链表头结点,会在链表的末尾插入新节点。
```c
void insert(struct node** head, datatype value) {
if (*head == NULL) {
*head = (struct node*)malloc(sizeof(struct node));
(*head)->data = value;
(*head)->next = NULL;
} else {
struct node* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = (struct node*)malloc(sizeof(struct node));
temp->next->data = value;
temp->next->next = NULL;
}
}
```
4. 读取元素:通过遍历链表,可以实现从头开始逐个读取节点的数据。
```c
datatype read(struct node* head) {
if (head == NULL) {
printf("链表为空.\n");
return '\0';
} else {
datatype result = head->data;
return result;
}
}
```
5. 删除元素:可以提供一个函数删除指定位置的节点,这里假设删除的是头节点的某个特定值。
```c
void deleteNode(struct node** head, datatype value) {
if (*head != NULL && (*head)->data == value) {
struct node* temp = *head;
*head = (*head)->next;
free(temp);
} else {
struct node* current = *head;
while (current->next != NULL && current->next->data != value) {
current = current->next;
}
if (current->next != NULL) {
struct node* toDelete = current->next;
current->next = current->next->next;
free(toDelete);
}
}
}
```
6. 主函数示例:在主函数中,你可以根据需要调用这些函数,例如创建、读取、插入、删除等操作。
```c
int main() {
// 初始化链表
struct node* list = createList();
// 添加操作...
insert(&list, 'A');
// 读取操作...
datatype data = read(list);
printf("读取的数据: %c\n", data);
// 删除操作...
deleteNode(&list, 'A');
// 等待用户选择其他操作...
return 0;
}
```
阅读全文
相关推荐















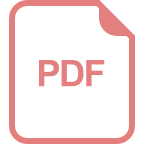
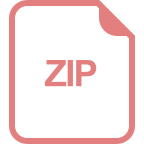
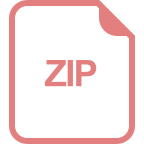
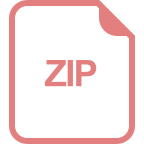