1-1 设链表的存储结构如下: typedef struct Node { Type data; //数据域;Type: 用户定义数据类型 struct Node *next; //指针域 } Node, *LinkList; 实现链表的基本操作。给出正确的c++代码
时间: 2024-09-23 18:09:03 浏览: 18
在C++中,我们可以使用上述链表的存储结构来实现一些基本操作,如创建链表、插入节点、删除节点以及遍历链表。以下是对应的C++代码:
```cpp
#include<iostream>
// 定义链表节点和链表指针类型
template<typename T>
struct Node {
T data; // 数据域
Node<T>* next; // 指向下一个节点的指针
};
// 链表的基本操作
template<typename T>
class LinkedList {
private:
Node<T>* head; // 链表头部
public:
// 构造函数,创建空链表
LinkedList() : head(nullptr) {}
// 插入节点到链表前端
void insertFront(T value) {
Node<T>* newNode = new Node<T>{value, head};
head = newNode;
}
// 插入节点到链表尾部
void insertEnd(T value) {
if (head == nullptr) {
head = new Node<T>{value, nullptr};
return;
}
Node<T>* current = head;
while (current->next != nullptr)
current = current->next;
current->next = new Node<T>{value, nullptr};
}
// 删除指定值的第一个匹配节点
void deleteNode(T value) {
if (head == nullptr || head->data == value)
head = head->next;
else {
Node<T>* current = head;
while (current->next != nullptr && current->next->data != value)
current = current->next;
if (current->next != nullptr)
current->next = current->next->next;
}
}
// 遍历链表并打印节点数据
void printList() {
Node<T>* temp = head;
while (temp != nullptr) {
std::cout << temp->data << " ";
temp = temp->next;
}
std::cout << "\n";
}
};
// 示例使用
int main() {
LinkedList<int> list;
list.insertFront(5);
list.insertEnd(10);
list.printList(); // 输出: 5 10
list.deleteNode(5);
list.printList(); // 输出: 10
return 0;
}
```
这个C++代码首先定义了一个模板类型的链表结构(Node),然后创建了一个LinkedList类,包含了插入、删除和打印链表的操作。在main函数中展示了如何创建和操作链表。
相关推荐
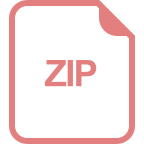
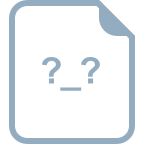
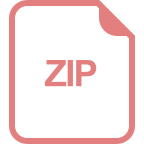
















