2.定义一个包含学生信息(学号,姓名,成绩)的单链表,使其具有如下功能:(选做)(1)根据指定学生个数,逐个输入学生信息;(2)逐个显示学生表中所有学生的相关信息;(3)根据姓名进行查找,返回此学生的
时间: 2024-09-25 15:04:17 浏览: 45
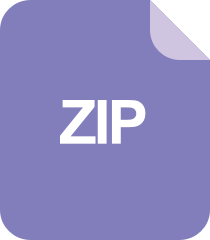
数据结构:定义一个包含学生信息(学号、姓名、成绩)的顺序表_数据结构

要定义一个包含学生信息的单链表结构,并实现上述功能,你可以按照以下步骤进行:
1. 定义节点(Node)类,用于存储学生的学号、姓名和成绩:
```java
public class Student {
int studentId;
String name;
double score;
public Student(int id, String n, double s) {
this.studentId = id;
this.name = n;
this.score = s;
}
// getters and setters for the fields
}
```
2. 定义链表(StudentList)类,包含链表的头结点以及插入、查找等操作:
```java
public class StudentLinkedList {
private Node head; // 链表头结点
// 插入学生信息的方法
public void addStudent(Student student) {
if (head == null) {
head = new Node(student);
} else {
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = new Node(student);
}
}
// 显示所有学生信息的方法
public void displayStudents() {
Node current = head;
while (current != null) {
System.out.println("学号:" + current.studentId +
", 姓名:" + current.name +
", 成绩:" + current.score);
current = current.next;
}
}
// 根据姓名查找学生的方法
public Student findStudentByName(String name) {
Node current = head;
while (current != null) {
if (current.name.equals(name)) {
return current;
}
current = current.next;
}
return null; // 如果未找到则返回null
}
}
```
阅读全文
相关推荐
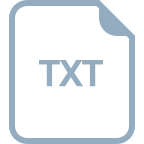
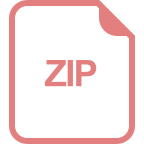















