Python实现ICA算法代码
时间: 2023-11-05 09:19:36 浏览: 89
Sure! Here is an example of Python code for implementing the Independent Component Analysis (ICA) algorithm using the FastICA approach:
```python
import numpy as np
from scipy import linalg
def ica(X, n_components, max_iter=1000, tol=1e-4):
n_samples, n_features = X.shape
# Centering the data
X_mean = X.mean(axis=0)
X -= X_mean
# Whitening the data
_, S, V = linalg.svd(X, full_matrices=False)
X_rotated = np.dot(X, V.T[:n_components])
# Initializing unmixing matrix W
W = np.random.rand(n_components, n_components)
for iter_ in range(max_iter):
W_old = W.copy()
# Deflating the rows of W
for i in range(n_components):
w = W[i, :].copy().reshape((1, -1))
w_dot_x = np.dot(w, X_rotated.T)
w_dot_w = np.dot(w, W.T)
# Updating the weights using neg-entropy as contrast function
g = np.tanh(w_dot_x).flatten()
g_dot_x = np.dot(g, X_rotated)
g_dot_g = np.dot(g, g.T)
W[i, :] = (g_dot_x - g_dot_g * w.flatten()) / n_samples
# Decorrelating the weight vector
W[i, :] = W[i, :] - np.dot(np.dot(W[:i], W[i].T), W[:i])
# Normalizing the weight vector
W[i, :] /= np.sqrt(np.sum(W[i, :] ** 2) + 1e-10)
# Checking for convergence
if np.max(np.abs(np.abs(np.diag(np.dot(W, W_old.T))) - 1)) < tol:
break
# Reconstructing the sources
S = np.dot(X_rotated, W.T) + X_mean
return S
```
To use this code, you can provide your data matrix `X` as input, along with the desired number of independent components `n_components`. The `max_iter` and `tol` parameters control the maximum number of iterations and convergence tolerance for the algorithm, respectively. The function will return the estimated independent components `S`.
Note that this code assumes that your data matrix `X` has shape `(n_samples, n_features)`, where each row represents a sample and each column represents a feature. Make sure you have imported the necessary libraries like `numpy` and `scipy` before running this code.
I hope this helps you with implementing the ICA algorithm in Python! Let me know if you have any further questions.
相关推荐
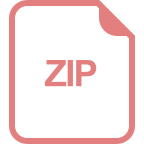














