在自定义点云类型中添加rgb并注册
时间: 2024-09-09 09:05:20 浏览: 42
在自定义点云类型中添加RGB信息并进行注册,通常需要通过编程语言和相关库来实现。以C++语言结合PCL(Point Cloud Library)为例,可以按照以下步骤进行:
1. 定义点云数据结构:首先定义一个点云数据结构,这个结构体通常继承自PCL中的`pcl::PointNormal`或`pcl::PointXYZ`等基础点云类型,然后添加RGB信息,通常是三个浮点数表示红色、绿色和蓝色的值。
2. 创建点云对象:根据定义的结构体创建一个点云对象,这个对象可以是一个包含RGB信息的点云数组。
3. 点云注册:点云注册通常是指找到两个点云之间的对应关系,即匹配它们相似的特征点。可以使用诸如迭代最近点(ICP)算法来对点云进行配准。如果点云中包含了RGB信息,可以利用颜色信息来帮助提高匹配的准确度。
4. 执行算法:使用PCL提供的算法对点云进行配准,得到变换矩阵,然后使用这个矩阵来变换一个点云,使它与另一个点云对齐。
5. 结果展示:最后,展示或者使用配准后的点云数据。
以下是一个简单的代码示例,展示如何在PCL中定义一个包含RGB信息的点云,并使用ICP算法进行注册:
```cpp
#include <pcl/point_types.h>
#include <pcl/io/pcd_io.h>
#include <pcl/registration/icp.h>
// 定义一个包含RGB信息的点云类型
struct PointTypeWithColor
{
float x, y, z;
uint32_t rgb;
};
// 将RGB信息的三个通道分离出来
uint8_t r = (point.rgb & 0x00ff0000) >> 16;
uint8_t g = (point.rgb & 0x0000ff00) >> 8;
uint8_t b = (point.rgb & 0x000000ff);
// 读取点云数据
pcl::PointCloud<PointTypeWithColor> cloud_source, cloud_target;
if (pcl::io::loadPCDFile<PointTypeWithColor>("source.pcd", cloud_source) == -1 ||
pcl::io::loadPCDFile<PointTypeWithColor>("target.pcd", cloud_target) == -1)
return -1;
// ICP算法注册点云
pcl::IterativeClosestPoint<PointTypeWithColor, PointTypeWithColor> icp;
icp.setInputSource(cloud_source.makeShared());
icp.setInputTarget(cloud_target.makeShared());
// 执行ICP算法,得到配准后的点云
pcl::PointCloud<PointTypeWithColor> output_cloud;
icp.align(output_cloud);
// 检查配准是否成功并输出结果
if (icp.hasConverged())
{
std::cout << "ICP has converged, score is " << icp.getFitnessScore() << std::endl;
std::cout << "ICP transformation " << icp.getFinalTransformation() << std::endl;
}
```
阅读全文
相关推荐
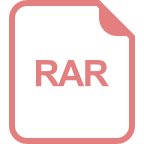
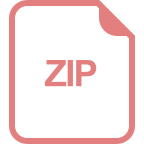
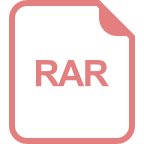















