用vhdl设计一个数字频率计 基本功能: 1)用同步脉冲检测随机输入信号的变化次数,2)用一个标准时钟计算输入信号的频率,3)采样周期设为0.5秒: 4)输入信号可用单脉冲按钮模拟。
时间: 2024-12-18 14:23:09 浏览: 8
在VHDL(Verilog High-Level Design Language)中设计一个数字频率计可以分为几个步骤:
1. **数据类型和模块声明**:
首先,需要定义一些数据类型,如整型用于存储计数次数和频率,以及状态机的状态等。开始时创建一个名为"FrequencyCounter"的实体和结构体。
```vhdl
entity FrequencyCounter is
Port (
clk : in std_logic;
input_signal : in std_logic;
sample_period : constant time := 500 ns; -- 0.5 秒的采样周期
button : in std_logic -- 单脉冲按钮输入
);
end FrequencyCounter;
```
2. **状态机设计**:
使用有限状态机(FSM)来处理计数和频率计算。通常有起始状态、计数状态、测量频率状态和结束状态。当同步脉冲`clk`上升沿到来且`button`按下时,从起始状态进入计数状态。
```vhdl
architecture Behavioral of FrequencyCounter is
type States is (Idle, Counting, Measuring, Done);
signal current_state : States;
signal count : integer range 0 to 999999; -- 可能的最大变化次数
begin
process(clk, button)
begin
if rising_edge(clk) then
case current_state is
when Idle =>
if button = '1' then
current_state <= Counting;
end if;
when Counting =>
if input_signal = '1' and input_signal'event then
count <= count + 1;
if count = 999999 then
current_state <= Measuring;
end if;
end if;
when Measuring =>
if rising_edge(clk) then
frequency <= count / sample_period;
current_state <= Done;
end if;
when Done =>
-- Do nothing, just wait for new measurement or reset
end case;
end if;
end process;
end Behavioral;
```
3. **输出和外部连接**:
设计好状态机后,你需要将频率结果输出到适当的地方,比如LED显示或者通过串口输出。你可以添加一个信号`frequency_out`来传递计算出的频率。
```vhdl
signal frequency_out : real;
...
Port (
...
freq_out : out frequency_out;
...
);
```
4. **完整设计文件**:
最后,在顶层实体中包含并配置这个模块,并提供所有必要的端口连接。记得添加适当的包声明、库引用和其他必要的部分。
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
entity Top_Level is
generic(
ClockPeriod : time := 10 ns); -- 根据实际晶振设置时钟周期
Port (
clk : in std_logic;
input_signal : in std_logic;
button : in std_logic;
freq_out : out real
);
end Top_Level;
architecture Behavioral of Top_Level is
component FrequencyCounter is
Port (
clk : in std_logic;
input_signal : in std_logic;
button : in std_logic;
freq_out : out real
);
end component;
signal local_clk : std_logic;
signal local_button : std_logic;
begin
local_clk <= not clk; -- 驱动计数器的反相时钟
local_button <= button; -- 复制按钮输入
counter : FrequencyCounter
port map (
clk => local_clk,
input_signal => input_signal,
button => local_button,
freq_out => freq_out
);
end Behavioral;
```
阅读全文
相关推荐





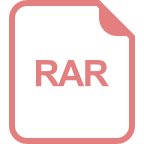










